Django Meets Docker: Simplifying Your Web Development Workflow
Web development has come a long way in the past decade. Technologies like Django, a high-level Python web framework, have made it easier to develop highly scalable, secure, and robust web applications. As projects become more complex, teams often choose to hire Django developers to ensure their applications are in expert hands. However, every developer, regardless of their expertise, knows the hassle of setting up a consistent development environment. One wrong configuration can lead to hours of debugging, and it becomes even more complicated when trying to maintain parity between the production and development environments. This is where Docker, an open-source platform used for developing, shipping, and running applications, steps in, providing a solution that ensures consistency and efficiency across the development process.
What is Docker?
Docker is an open-source containerization platform. It enables developers to package applications into containers—standardized executable components that combine application source code with the operating system (OS) libraries and dependencies required to run that code in any environment. In simple terms, Docker can help you “build once, run anywhere.”
Why Docker for Django?
While Django simplifies web development, Docker complements Django by abstracting the environment setup and making your application run uniformly, irrespective of the host system. The benefits of Docker are:
- Consistency across multiple development environments.
- Isolation of applications – each container is independent.
- Easier debugging process with Docker’s built-in tools.
- Less overhead than traditional virtual machines.
Now, let’s walk through the process of containerizing a Django web application using Docker.
Setting Up a Django Project
Let’s assume you have a basic Django application set up. If not, you can quickly create a new Django project using the following commands:
```bash # Install Django pip install Django # Create a new Django project django-admin startproject mysite # Navigate into the project cd mysite # Start the Django server python manage.py runserver ```
Once you’ve done that, navigate to `http://localhost:8000` in your browser, and you should see the Django welcome page.
Dockerizing the Django Application
To dockerize our Django application, we will require two files: `Dockerfile` and `docker-compose.yml`.
Dockerfile
The Dockerfile is a text file that contains the instructions needed to create a new Docker image. For our Django project, it might look like this:
```Dockerfile # Pull the official base image FROM python:3.8-slim-buster # Set environment variables ENV PYTHONDONTWRITEBYTECODE 1 ENV PYTHONUNBUFFERED 1 # Set work directory WORKDIR /code # Install dependencies COPY requirements.txt /code/ RUN pip install -r requirements.txt # Copy project COPY . /code/ ```
This Dockerfile does the following:
- Starts `FROM` the Python 3.8 base image.
- Sets two `ENV`ironment variables that prevent Python from writing pyc files to disc and buffers it to stdout.
- Set the `WORKDIR`ectory inside the container to `/code`.
- Copies the `requirements.txt` file into the container.
- Run `pip install` to install our application’s dependencies.
- Finally, it `COPY`s our Django application code into the container.
Docker Compose
Docker Compose is a tool that allows you to define and manage multi-container Docker applications. It uses a YAML file to specify the application’s services and with a single command, you can create and start all the services.
Our `docker-compose.yml` file could look like this:
```yaml version: '3.8' services: web: build: . command: python manage.py runserver 0.0.0.0:8000 volumes: - .:/code ports: - 8000:8000 ```
Here, we’re defining a single service “web”, which:
- Builds from the Dockerfile in the current directory.
- Run the command `python manage.py runserver 0.0.0.0:8000` upon launch.
- Maps our project’s directory to the `/code` directory in the Docker container.
- Forwards the exposed port 8000 on the container to port 8000 on our host machine.
Running the Dockerized Django Application
With our `Dockerfile` and `docker-compose.yml` ready, we can now bring up our Dockerized Django application using the following command:
```bash docker-compose up ```
Once your services are up, navigate to `http://localhost:8000` in your web browser. You should see your Django app running as expected, but now it’s running inside a Docker container!
Conclusion
In this blog post, we’ve covered the basics of using Docker with Django, providing you with the knowledge needed to create a containerized development environment. If you’re looking to scale up your projects, hiring Django developers could be a strategic move. As your Django app grows, you might want to add more services such as a database or a caching system to your Docker Compose file. Docker and Django, when harnessed by skilled developers, make a powerful duo for developing and deploying robust web applications. Happy coding!
Table of Contents
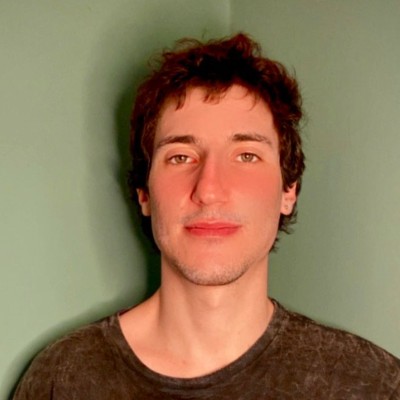
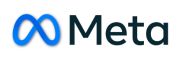