Mastering Django Admin: Your Guide to Efficient Web Application Management
Django, a popular Python web framework, has become the go-to choice for developers, and the preferred option for businesses looking to hire Django developers. It offers robustness, scalability, and comes with many in-built features, making it suitable for both small and large projects alike. One of the many powerful features Django provides is the Django Admin – a ready-to-use user interface for administrative activities.
The Django Admin isn’t just a mere add-on but an extensively powerful tool that allows developers to interact with their database schema and data directly. Its comprehensive capabilities are why businesses hire Django developers for optimal web solutions. For those who aren’t familiar with Django Admin, you may wonder why it’s worth learning. The answer lies in its capacity to accelerate development times, automate repetitive tasks, and provide a user-friendly interface for data management. In this blog, we’ll delve into the workings of Django Admin and discuss its potential by walking through some practical examples.
Getting Started With Django Admin
To start using Django Admin, first, you’ll need to set up a Django project. Once you have a Django project and an app, register your models in the admin.py file of your app.
```python from django.contrib import admin from .models import Blog admin.site.register(Blog) ```
After registering your models, you can start the server, and visit http://localhost:8000/admin. Django will present you with a login page. By default, Django Admin requires users to log in for security purposes.
Creating a Superuser
To access Django Admin, you’ll need to create a superuser, who has all permissions and can create, edit, and delete records. Here’s how to do it:
```shell python manage.py createsuperuser ```
After running the command, provide a username, email, and password as prompted.
Customizing Django Admin
The power of Django Admin lies in its flexibility. You can customize how your data is displayed, interacted with, and updated. Let’s look at some customization examples.
Example 1: Changing Displayed Fields
Suppose you have a model `Blog` with the fields `title`, `author`, `created_at`, `updated_at`, and `content`. By default, the Django Admin interface shows only the `str` representation of each instance.
```python class Blog(models.Model): title = models.CharField(max_length=200) author = models.ForeignKey(User, on_delete=models.CASCADE) created_at = models.DateTimeField(auto_now_add=True) updated_at = models.DateTimeField(auto_now=True) content = models.TextField() def __str__(self): return self.title ```
To change this and display more fields, you can customize the `BlogAdmin` as follows:
```python class BlogAdmin(admin.ModelAdmin): list_display = ('title', 'author', 'created_at', 'updated_at') admin.site.register(Blog, BlogAdmin) ```
Example 2: Filter Options
Django Admin allows us to add filter options. For instance, if you want to filter blogs based on authors or creation time, update `BlogAdmin`:
```python class BlogAdmin(admin.ModelAdmin): list_display = ('title', 'author', 'created_at', 'updated_at') list_filter = ('author', 'created_at') admin.site.register(Blog, BlogAdmin) ```
Example 3: Search Functionality
You can enable search functionality in Django Admin. Here, let’s add a search box to search blogs by title:
```python class BlogAdmin(admin.ModelAdmin): list_display = ('title', 'author', 'created_at', 'updated_at') list_filter = ('author', 'created_at') search_fields = ('title',) admin.site.register(Blog, BlogAdmin) ```
Advanced Customization
While the basic customization options can be handy, Django Admin offers a lot more. Here are some advanced examples:
Example 4: Custom Actions
Django Admin lets you perform actions on multiple records simultaneously. Let’s add a custom action to mark selected blogs as featured:
```python class BlogAdmin(admin.ModelAdmin): ... actions = ['mark_as_featured'] def mark_as_featured(self, request, queryset): queryset.update(is_featured=True) mark_as_featured.short_description = 'Mark selected blogs as Featured' admin.site.register(Blog, BlogAdmin) ```
Example 5: Inline Editing
Inline editing allows you to add or edit related models on the same page as the parent model. This can be helpful if you have models related through `ForeignKey`, `OneToOne`, or `ManyToMany` relations. For instance, if we have `Comment` model related to `Blog`, you can edit them inline:
```python class CommentInline(admin.TabularInline): model = Comment class BlogAdmin(admin.ModelAdmin): ... inlines = [CommentInline] admin.site.register(Blog, BlogAdmin) ```
Conclusion
Django Admin, a built-in feature of Django, provides developers with a powerful interface for managing web applications. Its strength lies in its flexibility, which is why many businesses choose to hire Django developers for their projects. Django Admin can be customized to fit a variety of project needs, from basic model registration and field display to more complex actions like inline editing and bulk actions. This versatility makes it a crucial tool in a Django developer’s arsenal, saving significant development time and simplifying numerous administrative tasks. However, while Django Admin is a potent tool, it is crucial to handle it with care, especially in a production environment, due to its full access nature.
Table of Contents
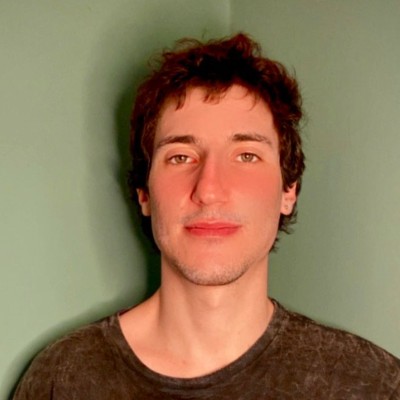
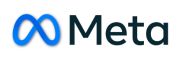