Take Your Django App to the Next Level: Email Sending & Receiving Simplified
Django, a popular high-level Python web framework, promotes rapid development and clean, pragmatic design. One of the most frequently needed functionalities in web applications is the capability to send and receive emails. Whether you are verifying a new user’s email, sending out newsletters, or even creating a system to process emails, Django has you covered. In this post, we’ll explore how to integrate email functionalities into your Django application, both for sending and receiving emails.
Table of Contents
1. Sending Emails with Django
1.1 Setting up Email Backends
Before you can send emails, you’ll need to configure Django with your email service details. Django provides various email backends. The most commonly used is the SMTP email backend.
In your `settings.py`:
```python EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend' EMAIL_HOST = 'your-smtp-server.com' # e.g., 'smtp.gmail.com' for Gmail's SMTP server EMAIL_PORT = 587 EMAIL_USE_TLS = True EMAIL_HOST_USER = 'your@email.com' EMAIL_HOST_PASSWORD = 'your-email-password' ```
Replace placeholders with your SMTP server details.
1.2 Sending a Simple Email
Now, you’re ready to send emails:
```python from django.core.mail import send_mail send_mail( 'Subject here', 'Here is the message.', 'from@example.com', ['to@example.com'], fail_silently=False, ) ```
1.3 Using Email Templates
For sending rich HTML emails or if you require a template:
- First, create an HTML template in your templates directory, e.g., `email_template.html`.
- Then, render this template and send it:
```python from django.template.loader import render_to_string from django.core.mail import EmailMessage message_content = render_to_string('email_template.html', {'name': 'John'}) email = EmailMessage( 'Your Subject Here', message_content, 'from@example.com', ['to@example.com'] ) email.content_subtype = 'html' email.send() ```
2. Receiving Emails in Django
While Django itself doesn’t provide tools to receive emails, you can leverage third-party tools and integrate them into your Django application. Here are two common approaches:
2.1. Using a Webhook:
Services like SendGrid, Mailgun, and others offer webhooks to notify your application when an email is received. Here’s a generic way of setting this up:
- Create a Django view to handle the webhook POST request.
- Configure the third-party service to hit this endpoint when an email is received.
```python # views.py from django.http import JsonResponse from django.views.decorators.csrf import csrf_exempt @csrf_exempt def handle_incoming_email(request): if request.method == 'POST': sender = request.POST.get('sender') subject = request.POST.get('subject') body = request.POST.get('body') # Process the email (e.g., store in the database, trigger some action, etc.) return JsonResponse({'status': 'success'}) return JsonResponse({'status': 'invalid request'}, status=400) ```
- Add this view to your `urls.py`.
2.2. Polling an IMAP or POP3 Mailbox:
For services that don’t offer webhook integration, another approach is to periodically poll an email inbox using IMAP or POP3.
Here’s a basic example using Python’s `imaplib`:
```python import imaplib MAIL_SERVER = 'your-imap-server.com' MAIL_PORT = 993 MAIL_USERNAME = 'your@email.com' MAIL_PASSWORD = 'your-email-password' def check_for_emails(): mailbox = imaplib.IMAP4_SSL(MAIL_SERVER, MAIL_PORT) mailbox.login(MAIL_USERNAME, MAIL_PASSWORD) mailbox.select('inbox') result, data = mailbox.search(None, 'ALL') if result == 'OK': for num in data[0].split(): result, email_data = mailbox.fetch(num, '(RFC822)') raw_email = email_data[0][1] # Parse and process the email content ```
For better email parsing, consider using Python libraries like `email` or `beautifulsoup4`.
Conclusion
Integrating email functionality into your Django application unlocks a plethora of opportunities, ranging from user notifications to sophisticated workflows based on email content. By setting up Django’s email backends for sending and using webhooks or IMAP/POP3 polling for receiving, you can seamlessly embed email-related features into your web app.
Whether it’s a basic registration confirmation, password reset, or a complex task like parsing received emails for data extraction, Django’s flexibility combined with Python’s rich ecosystem offers a powerful solution. Remember, always test your email functionalities thoroughly to ensure consistent and reliable performance.
Table of Contents
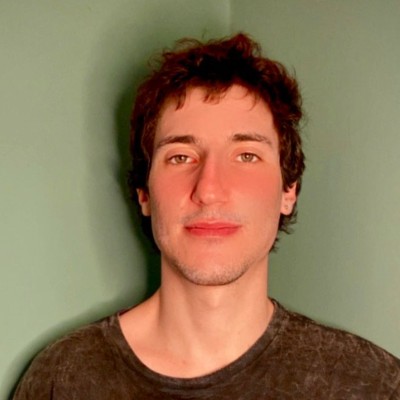
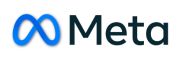