How to implement a file download feature in Django REST framework?
To implement a file download feature in Django REST framework, you’ll need to create an API endpoint that allows clients to request and download files. Here’s a step-by-step guide on how to achieve this:
- Setup Your Django Project:
Ensure you have a Django project set up with Django REST framework installed. If you haven’t already, create a Django app where you’ll implement the file download feature.
- Prepare the File:
First, you need to prepare the file you want to make available for download. This could be an image, document, or any other type of file. Store the file in a directory within your project’s file system or, for better scalability, consider using cloud storage like AWS S3.
- Create a Serializer:
In your Django app, create a serializer using Django REST framework’s serializers. If you’re storing file paths in your database models, you can create a serializer that includes the file field.
- Create a View:
Next, create a view that handles the file download logic. You can use a generic view like `RetrieveAPIView` if you’re fetching the file based on an object’s ID. In this view, you’ll define the logic for retrieving and serving the file.
- Configure URLs:
Configure the URL routing in your Django app to map the view to a URL endpoint. This URL should be where clients will make GET requests to initiate the download.
- Implement the Download Logic:
In your view, implement logic to fetch the file, open it in binary read mode, and then use Django’s `FileResponse` to serve the file as a downloadable attachment. Set appropriate response headers, such as `Content-Disposition`, to specify the filename and content type.
- Secure Access (Optional):
Depending on your application’s requirements, you might want to secure access to the file download endpoint. You can implement authentication and authorization checks to ensure that only authorized users can download files.
- Testing:
Test your file download feature using tools like Postman or cURL. Ensure that files are downloaded correctly, and the response headers are set as expected.
- Documentation:
Update your API documentation to inform clients about the new file download endpoint, including its URL and any authentication requirements.
- Deployment and Scalability:
Finally, deploy your Django application to your production server. If you expect heavy download traffic, consider using a content delivery network (CDN) or cloud storage to efficiently serve files.
By following these steps, you can successfully implement a file download feature in Django REST framework, allowing clients to access and download files from your API.
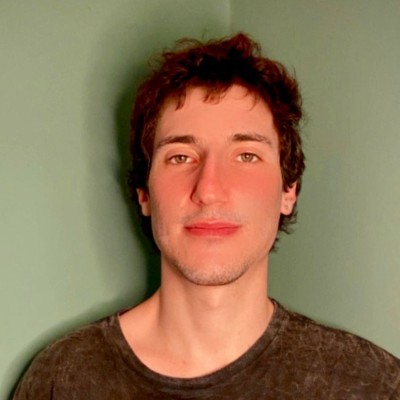
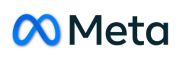