How to handle file uploads in Django using Amazon S3 or other cloud storage providers?
Handling file uploads in Django and storing them on cloud storage providers like Amazon S3 is a common practice for scalability, durability, and cost-effectiveness. Here’s a step-by-step guide on how to achieve this:
- Install Required Packages:
Start by installing the necessary packages for cloud storage support and Django’s file handling:
```bash pip install django-storages boto3 ```
- Configure Django Settings:
In your Django project’s settings, configure the storage backend and add the necessary credentials for your cloud storage provider. Here’s an example for Amazon S3 using the `boto3` library:
```python # settings.py AWS_ACCESS_KEY_ID = 'your-access-key-id' AWS_SECRET_ACCESS_KEY = 'your-secret-access-key' AWS_STORAGE_BUCKET_NAME = 'your-bucket-name' AWS_S3_REGION_NAME = 'your-region-name' DEFAULT_FILE_STORAGE = 'storages.backends.s3boto3.S3Boto3Storage' ```
Replace the placeholders with your actual AWS credentials and bucket name.
- Modify Your Model:
In the model where you want to handle file uploads, add a `FileField` or `ImageField` like this:
```python from django.db import models class YourModel(models.Model): upload = models.FileField(upload_to='uploads/') ```
The `upload_to` parameter specifies the folder structure within your cloud storage bucket.
- Update Your Forms and Views:
Ensure your forms and views are set up to handle file uploads as usual. When a user submits a file through a form, Django will automatically store it on your configured cloud storage.
- Serving Media Files:
To serve media files (user-uploaded files) during development, add the following to your project’s `urls.py`:
```python from django.conf import settings from django.conf.urls.static import static urlpatterns = [ # ... ] if settings.DEBUG: urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT) ```
This serves media files directly from your cloud storage when debugging.
With these steps, you can handle file uploads in Django and store them on cloud storage providers like Amazon S3. This approach allows you to take advantage of the scalability and reliability of cloud storage while seamlessly integrating it into your Django project.
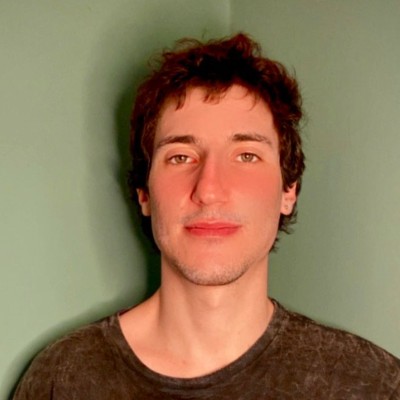
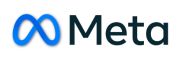