What are Django Forms, and how to create and handle them?
Django forms are a key component of web development, providing an efficient way to handle HTML forms and user input in Django applications. They simplify the process of collecting, validating, and processing data submitted by users. Here’s an overview of what Django forms are and how to create and handle them:
What Are Django Forms?
Django forms are Python classes that define the structure and behavior of HTML forms on your website. They allow you to create and manipulate web forms easily, including handling data validation, rendering form fields, and processing user input. Forms are an essential part of building interactive web applications and collecting information from users in a structured manner.
Creating and Handling Django Forms:
- Form Class Creation: To create a Django form, define a Python class that inherits from `django.forms.Form`. In this class, you define the form’s fields using various field types (e.g., `CharField`, `EmailField`, `IntegerField`) and specify validation rules and widgets.
- Rendering Forms in Templates: To display a form in your HTML template, use Django’s built-in template tags like `{% for %}` and `{% csrf_token %}` to loop through form fields and include a security token, which protects against cross-site request forgery (CSRF) attacks.
- Form Submission Handling: In your Django view, you can handle form submissions by checking the request method (POST or GET). When a user submits a form, you typically process the data, validate it, and take appropriate actions. Use the `request.POST` dictionary to access the submitted form data. If the form is valid, save or update data as needed.
- Form Validation: Django forms provide automatic data validation. Define validation rules in your form class using field-specific attributes like `required`, `min_length`, or custom validation methods. Invalid form submissions are easy to detect and can be handled by returning the form with error messages to the user.
- Redirect After Submission: After processing a form submission, it’s a good practice to redirect the user to a success page or another relevant URL. This helps prevent accidental form resubmissions and keeps the user flow organized.
- Using Model Forms: If you need to create a form that corresponds to a model in your database, you can use Django’s `ModelForm`. This form class is automatically generated based on a model’s fields, simplifying the process of creating and updating model instances.
- Customizing Form Rendering: Django forms provide flexibility for customizing form rendering in templates. You can manually render each form field or use Django’s `{% form %}` template tag to render the entire form. Customizing the form’s appearance and behavior can be achieved through HTML, CSS, and JavaScript.
Django forms are a powerful tool for handling user input in web applications. They streamline the process of creating, rendering, and processing forms, making it easier to collect and validate data from users. By following Django’s form-handling conventions, you can build interactive and user-friendly web applications efficiently.
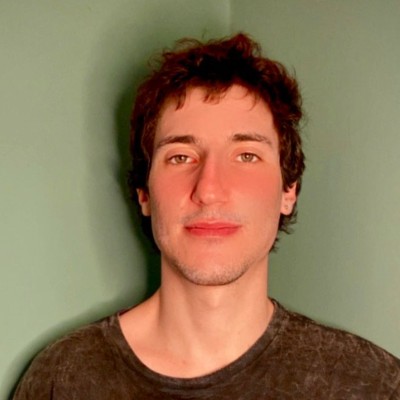
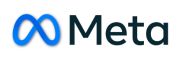