How to implement full-text search?
Implementing full-text search in Django can greatly enhance the search capabilities of your web application, allowing users to find relevant information quickly and efficiently. One popular way to achieve this is by using PostgreSQL’s full-text search capabilities along with Django’s `django.contrib.postgres` module. Here’s a step-by-step guide on how to implement full-text search in Django:
- Install PostgreSQL:
Ensure you have PostgreSQL installed and configured for your Django project. You can use the `psycopg2` library as the database adapter.
```bash pip install psycopg2-binary ```
- Create a PostgreSQL Full-Text Search Index:
In your database, create a full-text search index on the columns you want to search. This can be done using Django’s migration system or directly in your PostgreSQL database.
```python from django.contrib.postgres.indexes import GinIndex class YourModel(models.Model): # Your model fields # Add a text field for full-text search search_vector = SearchVectorField(null=True, blank=True) # Create a GIN index for the search_vector field class Meta: indexes = [GinIndex(fields=['search_vector'])] ```
- Update the Search Vector:
Populate the `search_vector` field with data from the fields you want to search. You can do this in your model’s `save()` method or by using database triggers.
```python from django.db import connection from django.db.models.signals import pre_save from django.dispatch import receiver from django.db.models import F from django.contrib.postgres.search import SearchVector @receiver(pre_save, sender=YourModel) def update_search_vector(sender, instance, **kwargs): instance.search_vector = ( SearchVector('field1', 'field2', 'field3', ...) # Fields to search ) ```
- Perform Full-Text Search:
To perform a full-text search, you can use Django’s `SearchQuery` and `SearchRank` classes in your views or queries:
```python from django.contrib.postgres.search import SearchQuery, SearchRank query = SearchQuery('search term') results = YourModel.objects.annotate(rank=SearchRank(F('search_vector'), query)).filter(rank__gte=0.3).order_by('-rank') ```
This query will return results sorted by relevance based on the full-text search.
- Display Search Results:
Finally, you can display the search results in your templates, providing users with a powerful full-text search experience.
By following these steps, you can implement full-text search in Django using PostgreSQL’s robust capabilities. This approach allows you to provide efficient and relevant search functionality to your users, making it easier for them to find the information they need within your application.
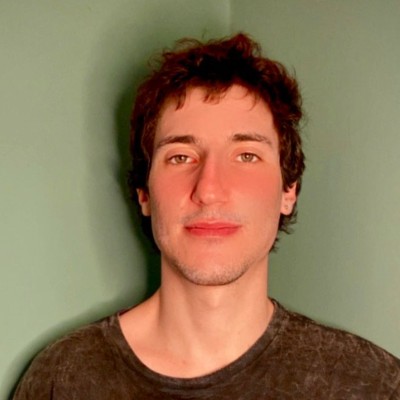
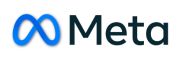