Ensuring Privacy in Django: A Developer’s Roadmap to GDPR
In the digital era, where data is the new gold, protecting user privacy has become paramount. The General Data Protection Regulation (GDPR), implemented in the EU, stands as a testament to this reality, setting a high standard for data protection and privacy. For developers and businesses using Django, a high-level Python web framework, aligning with GDPR is not just a legal requirement but also a commitment to user trust and safety. This article delves into how Django can be utilized to ensure GDPR compliance, providing practical examples and valuable external resources. You can hire Django Developers for your projects to ensure greater success.
Table of Contents
1. Understanding GDPR
Before diving into the technicalities, it’s crucial to understand the essence of GDPR. GDPR aims to empower individuals regarding their personal data, ensuring that data is collected, processed, and stored under strict conditions and for legitimate purposes. It also gives individuals the right to access, correct, and even erase their personal data. Compliance is mandatory for any business, regardless of location, that processes the data of EU citizens.
2. Key Principles of GDPR
– Consent: Clear consent must be obtained before collecting personal data.
– Right to Access: Individuals have the right to access their personal data.
– Data Portability: Individuals can transfer their data from one service provider to another.
– Right to be Forgotten: Individuals can request the deletion of their personal data.
3. Django and GDPR
Django, renowned for its “batteries-included” philosophy, offers a robust foundation to build GDPR-compliant applications. Let’s explore some of the ways Django can be utilized to align with GDPR requirements.
3.1. Consent Management
Consent is a cornerstone of GDPR. Django can manage user consents efficiently through its models and forms.
Example:
Create a `Consent` model to track user consents. This model can store user IDs, consent types (e.g., marketing emails, data collection), and timestamps.
```python from django.db import models class Consent(models.Model): user = models.ForeignKey(User, on_delete=models.CASCADE) consent_type = models.CharField(max_length=100) given_on = models.DateTimeField(auto_now_add=True) ```
3.2. Data Encryption
Encrypting sensitive data is critical under GDPR. Django’s built-in support for secure password hashing and its compatibility with third-party encryption tools make it a strong candidate for creating secure applications.
Example:
Utilize Django’s `AbstractBaseUser` model and `get_user_model` method to ensure secure password management.
```python from django.contrib.auth.models import AbstractBaseUser class User(AbstractBaseUser): ... ```
3.3. Data Anonymization
Anonymizing data, especially in development and testing environments, helps in GDPR compliance. Django’s fixtures and third-party packages like `Faker` can be used to generate anonymized data.
Example:
Use Django’s `dumpdata` command with natural keys to create anonymized fixtures for testing.
3.4. Logging and Monitoring
Monitoring access to personal data is a requirement under GDPR. Django’s logging framework can be configured to log data access and modifications, ensuring a trail for audits.
Example:
Configure Django’s logging to track access to sensitive views and models.
```python LOGGING = { ... 'loggers': { 'django': { 'handlers': ['file'], 'level': 'INFO', 'propagate': True, }, }, } ```
3.5. User Data Portability
Under GDPR, users have the right to obtain their data in a machine-readable format. Django’s serialization framework can be used to export user data in formats like JSON or XML.
Example:
Create a view that allows users to download their data in JSON format.
```python from django.http import JsonResponse from django.core import serializers def export_user_data(request): user_data = serializers.serialize('json', [request.user, ]) return JsonResponse(user_data, safe=False) ```
3.6. The Right to be Forgotten
Implementing the right to be forgotten can be challenging but is essential under GDPR. Django’s ORM (Object-Relational Mapping) allows for the secure deletion of user data upon request.
Example:
Create a view that allows users to delete their accounts and related data.
4. External Resources for Further Reading
- Django’s Security Documentation: Offers comprehensive insights into security practices, including data protection relevant for GDPR compliance Django Security Documentation.
- GDPR Official Website: For an in-depth understanding of GDPR and its implications GDPR.eu.
- Python Software Foundation’s GDPR Guide: Provides a detailed guide for Python applications, including Django, to comply with GDPR Python GDPR Guide.
Conclusion
While GDPR compliance might seem daunting, frameworks like Django provide the tools and features necessary to make this process more manageable. By leveraging Django’s capabilities in consent management, data encryption, anonymization, logging, data portability, and implementing the right to be forgotten, developers can build applications that not only comply with GDPR but also demonstrate a commitment to protecting user privacy.
You can check out our other blog posts to learn more about Django. We bring you a complete guide titled Django and Accessibility: Building Inclusive Web Applications along with the Django and Scalability: Building Web Apps for High Traffic and Django Templates: Creating Dynamic and Responsive Web Pages which will help you understand and gain more insight into the Django programming language.
Table of Contents
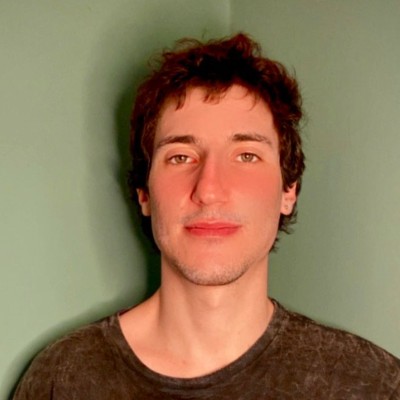
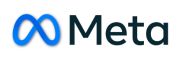