Navigate the World of Django Geolocation with Confidence
In the modern web application landscape, geolocation features have become increasingly popular. Whether it’s a food delivery service locating nearby restaurants, a travel platform suggesting attractions, or a social app connecting people in close proximity, location-based functionalities add significant value to an app’s user experience.
Table of Contents
Django, being a robust and versatile web framework, offers a suite of tools and libraries that can simplify the addition of geolocation features. In this article, we will explore how you can leverage Django to add location-based features to your app.
Prerequisites
– Familiarity with Django.
– Django project set up.
1. GeoDjango: The Geospatial Extension for Django
[GeoDjango](https://docs.djangoproject.com/en/3.2/ref/contrib/gis/) is an integrated geospatial framework included with Django. It provides tools to work with spatial databases, perform spatial queries, and handle geographic data.
1.1. Setting up GeoDjango:
– First, you need to install the necessary geospatial libraries. This might differ depending on your OS.
– Next, add `’django.contrib.gis’` to your `INSTALLED_APPS` in the Django settings.
1.2. Creating a Model with Geospatial Fields:
Let’s say you’re building a restaurant finder app. Your `Restaurant` model might look something like this:
```python from django.contrib.gis.db import models class Restaurant(models.Model): name = models.CharField(max_length=100) location = models.PointField() # Geospatial field representing a point in space. address = models.CharField(max_length=300) def __str__(self): return self.name ```
2. Adding Geolocation to Your Forms
You can use `PointField` in your model forms, allowing users to input latitude and longitude for entities like restaurants.
```python from django import forms from .models import Restaurant class RestaurantForm(forms.ModelForm): class Meta: model = Restaurant fields = ['name', 'location', 'address'] ```
3. Querying Geospatial Data
One of the prime benefits of using GeoDjango is the ability to make geospatial queries. For example:
Find restaurants within a certain radius:
```python from django.contrib.gis.geos import Point from django.contrib.gis.db.models.functions import Distance user_location = Point(x=longitude, y=latitude) nearby_restaurants = Restaurant.objects.annotate( distance=Distance('location', user_location) ).filter(distance__lte=5000) # finds restaurants within 5 km. ```
4. Integrating with Front-end Libraries
To display locations on a map, you can integrate with front-end mapping libraries such as Leaflet or Google Maps.
Using Leaflet with Django:
– Include Leaflet’s CSS and JavaScript files in your template.
– Initialize a map and plot the locations. You can pass the location data from Django views to the template.
```html <!-- HTML code to include Leaflet's CSS and JS --> <div id="map"></div> <script> let map = L.map('map').setView([default_lat, default_lon], 13); L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png').addTo(map); // Assuming you passed `nearby_restaurants` from your Django view let locations = {{ nearby_restaurants|safe }}; locations.forEach(location => { L.marker([location.lat, location.lon]).addTo(map); }); </script> ```
5. Geocoding with Django
Geocoding refers to converting human-readable addresses into geographic coordinates (and vice versa). You can use APIs like Google Maps Geocoding API or libraries like `geopy` to achieve this.
Using `geopy` to geocode an address:
```python from geopy.geocoders import Nominatim def get_location_from_address(address): geolocator = Nominatim(user_agent="your_app_name") location = geolocator.geocode(address) return (location.latitude, location.longitude) if location else None ```
Conclusion
Django, especially with the help of GeoDjango, offers powerful tools to integrate geolocation features seamlessly into your app. By creating geospatial models, integrating with front-end mapping libraries, and utilizing geocoding services, you can enhance your app’s functionality and user experience manifold.
Remember, while geolocation can add tremendous value to your app, always respect user privacy. Always ask for user consent before accessing or storing location data, and provide options to opt-out.
Table of Contents
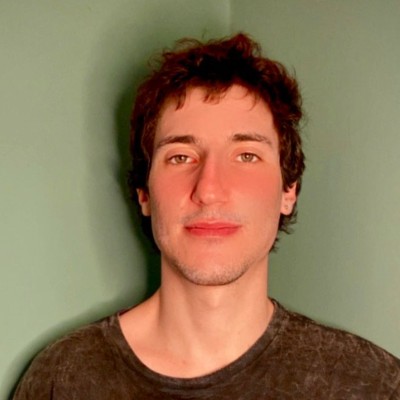
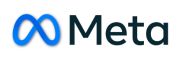