Mastering Django: A Comprehensive Guide to Web Development
Mastering Django is not a day’s task, but a journey. With a keen interest and consistent practice, you can develop the proficiency that leads companies to consistently hire Django developers for their projects. As you learn, grow, and begin to truly master this robust framework, you’ll find yourself in an elite league of professionals, the ones who can efficiently leverage Django’s rich ecosystem, robust architecture, and vibrant community to create web applications that stand out.
This expertise not only broadens your horizons but also becomes a strong reason for businesses to hire Django developers like you. Embrace Django, and step into the exciting world of web development!
Why Django?
Before we delve into the nuances of Django, it’s essential to comprehend why you might want to hire Django developers for your next project. Django follows the Don’t Repeat Yourself (DRY) principle and offers a clean and pragmatic design, which contributes to faster development. Its feature-packed toolkit, including an object-relational mapper (ORM), authentication support, and an automatically-generated admin interface, makes it a go-to choice for developers.
Setting Up Django
Assuming you have Python installed, setting up Django is as simple as running the pip command: `pip install Django`. Once installed, you can verify the Django installation using `django-admin –version`, which should display the installed Django version.
Creating Your First Django Project
Initiating a Django project is as straightforward as running `django-admin startproject myproject`, where “myproject” is the name of your project. This command will create a new directory named “myproject” with the necessary Django setup.
Understanding Django’s Project Structure
Once you hire Django developers, they will typically start by setting up the project structure. Let’s explore this structure:
– manage.py: This is a command-line utility that lets you interact with your project. It’s kind of a command center for your Django project.
– __init__.py: This is an empty file that informs Python that this directory should be considered a Python package.
– settings.py: This file includes configurations for your Django project.
– urls.py: This file includes URL declarations for the Django project.
– wsgi.py: This file serves as an entry-point for WSGI-compatible web servers to serve your project.
Creating an Application
Within a Django project, you can have multiple applications. Each application serves a specific purpose. Creating a Django application is as simple as running the command `python manage.py startapp myapp`, where “myapp” is your application’s name.
Models and Databases
Django uses models to represent database tables. Each model corresponds to a single table in the database. You can define a model in the `models.py` file of your application. For instance, a blog post model might look something like this:
```python from django.db import models class BlogPost(models.Model): title = models.CharField(max_length=200) content = models.TextField() pub_date = models.DateTimeField('date published')
Once you define your models, you can create a database schema using Django’s migration system. Run the command `python manage.py makemigrations myapp` followed by `python manage.py migrate` to apply these migrations to the database.
Admin Interface
One of the reasons why businesses hire Django developers is the powerful, automatically-generated admin interface Django provides. To activate the admin interface, edit your `admin.py` file like this:
```python from django.contrib import admin from .models import BlogPost admin.site.register(BlogPost)
Now you can add, delete, and edit your blog posts using the Django admin interface!
Views and URL Configuration
Views in Django handle the “what” — what data to send in response to a request. URLs handle the “where” — where to send a request. A simple view might look like this:
```python from django.http import HttpResponse from .models import BlogPost def index(request): latest_posts = BlogPost.objects.order_by('-pub_date')[:5] output = ', '.join([p.title for p in latest_posts]) return HttpResponse(output)
To wire this view to a URL, you would add the following to your application’s `urls.py` file:
```python from django.urls import path from . import views urlpatterns = [ path('', views.index, name='index'), ]
Testing in Django
Django comes with a built-in testing framework that encourages a test-driven development (TDD) approach. Once you hire Django developers, you can expect them to deliver bug-free applications by writing comprehensive tests.
Deploying Django Applications
Deployment is the stage where your application comes to life and is made accessible to users. This critical phase of development is simplified by Django’s compatibility with a wide array of servers, including Apache, NGINX, and others, and its easy integration with various cloud platforms such as AWS, GCP, and Heroku. It’s due to these conveniences in deployment and the comprehensive development capabilities of Django that companies actively hire Django developers. By employing these professionals, businesses ensure that their web applications not only have robust and efficient construction but also seamless deployment, making the entire process a breeze.
Conclusion
Django, a high-level Python web framework, empowers developers to break free from the redundant aspects of web development, propelling them toward the creation of robust, scalable, and maintainable applications with ease and efficiency. This proficiency and the ability to utilize Django’s vast functionalities are the reasons companies frequently hire Django developers to manage their web applications. As these developers can harness Django’s power to its fullest potential, they become invaluable assets in the web development landscape. In this blog, we will deep dive into Django to help you master this powerful tool and bolster your skills. This mastery not only increases your employability but also positions you as a sought-after resource for businesses looking to hire Django developers for their web application needs. Your journey to becoming a Django expert starts here!
Table of Contents
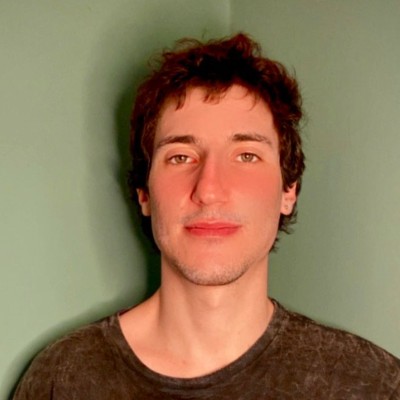
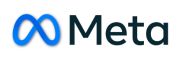