How to handle file uploads in Django REST framework?
Handling file uploads in Django REST framework (DRF) is a common requirement for building web APIs that accept and manage files, such as images, documents, or media. DRF provides a straightforward way to handle file uploads using its built-in `FileUploadParser` and serializers. Here’s how to do it:
- Serializer Configuration:
First, create a serializer that handles file uploads. Use the `serializers.FileField` or `serializers.ImageField` to define the fields for your file uploads. For example:
```python from rest_framework import serializers class FileUploadSerializer(serializers.Serializer): file = serializers.FileField() ```
- Configure the View:
In your DRF view, specify the serializer and the parser for file uploads. DRF includes a default `FileUploadParser`, so you don’t need to create one. Here’s an example view:
```python from rest_framework.parsers import FileUploadParser from rest_framework.response import Response from rest_framework.views import APIView class FileUploadView(APIView): parser_classes = (FileUploadParser,) def post(self, request): serializer = FileUploadSerializer(data=request.data) if serializer.is_valid(): # Access the uploaded file using serializer.validated_data['file'] # Process or save the file as needed return Response({'message': 'File uploaded successfully'}) else: return Response(serializer.errors, status=400) ```
- URL Configuration:
Define the URL route for your file upload view in your Django project’s URL configuration.
- Upload a File:
To upload a file, make a POST request to the file upload endpoint with the file attached as a form-data parameter named ‘file’. You can use tools like `curl`, Postman, or JavaScript libraries like Axios to perform the upload.
- Handling the File:
In the view’s `post` method, the uploaded file is accessible as `serializer.validated_data[‘file’]`. You can then process, save, or manipulate the file as needed.
- Error Handling:
Ensure you handle errors gracefully by checking if the serializer is valid and providing appropriate responses, including validation errors if the upload fails.
By following these steps, you can easily handle file uploads in Django REST framework, allowing your API to accept and manage files efficiently. This is particularly useful for building applications that require file storage and management capabilities, such as image or document hosting services.
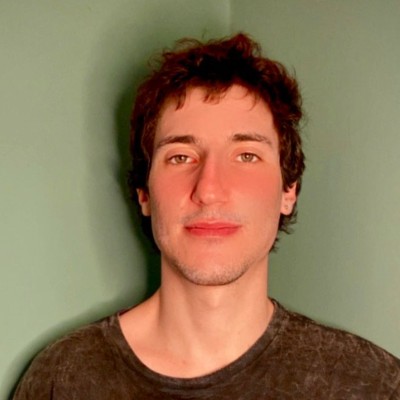
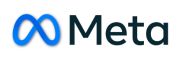