How to handle media files (uploads) in Django?
Handling media files, such as user uploads (e.g., images, documents, videos), in Django involves a different setup than static files. Django provides convenient tools to manage and serve media files in a web application. Here’s how to handle media files in Django:
- Directory Configuration: Start by configuring the directory where you want to store media files in your project’s `settings.py`. Define the `MEDIA_ROOT` setting, which specifies the absolute filesystem path to the directory where media files should be stored. For example:
```python MEDIA_ROOT = os.path.join(BASE_DIR, 'media') ```
- URL Configuration: Set the `MEDIA_URL` setting to specify the base URL for serving media files. By default, it’s set to `’/media/’`. Ensure that you have the following line in your project’s `urls.py` to serve media files during development:
```python from django.conf import settings from django.conf.urls.static import static if settings.DEBUG: urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT) ```
This configuration allows Django’s development server to serve media files during development.
- File Upload Field: In your Django models, when defining fields for file uploads (e.g., an image field), use the `models.FileField` or `models.ImageField` field type. For example:
```python from django.db import models class UserProfile(models.Model): profile_image = models.ImageField(upload_to='user_profiles/') ```
The `upload_to` parameter specifies the subdirectory within the `MEDIA_ROOT` where uploaded files will be stored.
- Form Handling: When handling file uploads in forms, use Django’s `forms.FileField` or `forms.ImageField` to create file input fields. Ensure that your form’s `enctype` is set to `’multipart/form-data’`.
- Template Usage: In your HTML templates, use the `url` attribute of the file field to display or link to uploaded media files. For example:
```html <img src="{{ user_profile.profile_image.url }}" alt="User Profile Image"> ```
- Security Considerations: Ensure that your web server is configured correctly to serve media files in production. It’s essential to secure user-uploaded files to prevent unauthorized access. Consider using access control mechanisms or restricting file types and sizes as needed.
By following these steps, you can effectively handle media files (uploads) in Django, allowing users to upload, display, and manage various types of content in your web application while ensuring proper storage and security.
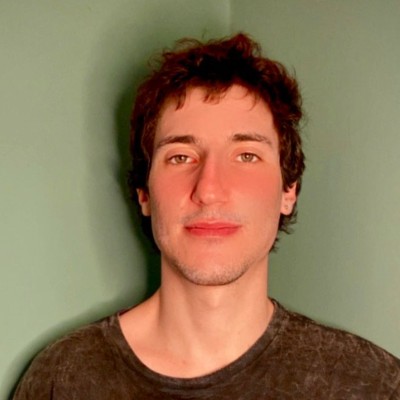
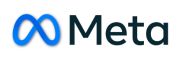