How to handle static files in Django?
Handling static files in Django is a crucial aspect of web development, as it ensures that your web application can serve static assets like CSS, JavaScript, images, and more efficiently. Django provides built-in tools to manage static files seamlessly. Here’s how to handle static files in Django:
- Directory Structure: Start by organizing your static files in a structured directory within your app or project. A common convention is to create a “static” directory at the app level or project level, like so:
``` myproject/ myapp/ static/ myapp/ css/ js/ img/ ```
- Configuration: In your project’s `settings.py`, make sure you have the following settings correctly configured:
– `STATIC_URL`: This setting defines the base URL for serving static files. By default, it’s set to `’/static/’`. You can change it if needed.
– `STATICFILES_DIRS`: Add the absolute paths to your app or project’s static directories to this list. For example:
```python STATICFILES_DIRS = [os.path.join(BASE_DIR, 'myapp/static')] ```
– `STATIC_ROOT`: Set this to an absolute filesystem path where you want Django to collect all static files during deployment. For example:
```python STATIC_ROOT = os.path.join(BASE_DIR, 'static') ```
- Collecting Static Files: Before deploying your application, run the following command to collect all static files into the `STATIC_ROOT` directory:
``` python manage.py collectstatic ```
This command copies all static files from your app’s static directories into the `STATIC_ROOT` directory, creating a single location for serving static files.
- Serving Static Files: During development, Django’s built-in development server automatically serves static files. In production, you should configure your web server (e.g., Nginx or Apache) to serve static files efficiently. You can also use a Content Delivery Network (CDN) for improved performance.
- Using Static Files in Templates: In your HTML templates, you can use the `{% static %}` template tag to generate URLs for your static files. For example:
```html <link rel="stylesheet" type="text/css" href="{% static 'myapp/css/style.css' %}"> ```
By following these steps, you can effectively handle static files in Django, ensuring that your web application serves them correctly both during development and production. Properly managing static files is essential for creating responsive and visually appealing web applications.
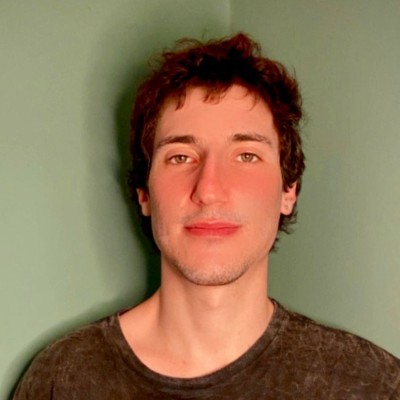
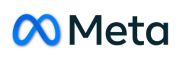