How to handle user notifications and emails in Django?
Handling user notifications and emails in Django is a crucial aspect of building web applications that engage and inform users effectively. Here’s a guide on how to handle these tasks:
- Set Up Email Configuration:
Django provides built-in support for sending emails. Start by configuring your email settings in your project’s `settings.py` file. You’ll need to specify email backends (e.g., SMTP, SendGrid) and credentials.
- Sending Emails:
To send emails, use Django’s `send_mail()` function or other email-related functions and classes. You can send plain text emails or use HTML templates to create rich email content.
- Email Templates:
Create email templates to maintain consistency in your email messages. Django allows you to use templates with the `render()` function to generate HTML content for emails.
- Notification System:
Implement a notification system to trigger and manage user notifications. You can use Django’s signals to detect events (e.g., a new message, a new follower) and send notifications accordingly.
- Message Queues:
For scalability and reliability, consider using message queues like Celery to handle email sending and notifications asynchronously. This ensures that your application remains responsive even when processing a large number of notifications or emails.
- Subscription Preferences:
Give users control over their notification preferences. Allow them to choose which types of notifications they want to receive and how frequently.
- Email Verification:
When users sign up or change their email address, implement email verification to confirm the authenticity of their email addresses. This helps prevent spam accounts and ensures accurate communication.
- Error Handling:
Implement error handling for email delivery failures. You can set up logging to monitor email delivery and handle exceptions gracefully.
- Testing:
Write tests for your email sending and notification logic to ensure they work correctly. You can use Django’s testing framework to automate this process.
- Third-Party Services:
Consider using third-party services like SendGrid, Mailgun, or AWS SES for email sending. They often provide robust delivery infrastructure and analytics.
- Security:
Ensure that sensitive information, such as email credentials and user data, is handled securely. Use environment variables or a secrets manager to store sensitive information.
- Optimization:
Optimize email content for different devices and email clients to ensure a consistent and appealing user experience.
- Analytics and Tracking:
Implement email tracking to monitor email open rates and click-through rates. This data can help you improve your email engagement strategies.
By following these steps and best practices, you can effectively handle user notifications and emails in your Django web application, providing users with a seamless and informative experience while maintaining the security and scalability of your email infrastructure.
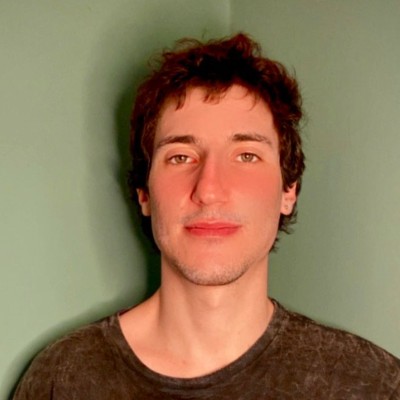
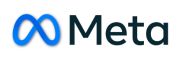