Django Q & A
How to implement real-time notifications in a Django application?
Implementing real-time notifications in a Django application can greatly enhance user engagement and provide timely updates. Here’s a high-level overview of how to achieve this:
- Choose a Real-Time Technology: To implement real-time notifications, you’ll need a technology that supports WebSocket communication. One popular choice is Django Channels, which extends Django to handle WebSocket connections.
- Install and Configure Django Channels: Start by installing Django Channels and configuring it in your Django project. You’ll need to add Channels to your project’s `settings.py` and set up routing to handle WebSocket connections.
- Create a Notification Model: Define a model to represent notifications in your application. This model should include fields like the recipient, message, timestamp, and any other relevant information.
- Implement Notification Logic: Write the logic for creating and sending notifications. This typically involves creating instances of the notification model when specific events occur in your application, such as when a user receives a new message or a comment is posted on their content.
- WebSocket Consumer: Create a WebSocket consumer that handles incoming WebSocket connections and manages real-time notifications. This consumer should listen for events and send notifications to connected clients when new notifications are created.
- Front-End Integration: On the client side, you’ll need to implement WebSocket connections using JavaScript. You can use libraries like Django Channels’ Channels-WebSocket or WebSocket libraries such as Socket.IO to handle this. When a user logs in, establish a WebSocket connection to the server.
- Displaying Notifications: Design and implement a user interface to display notifications to the user. This could be a notification dropdown, a real-time feed, or a dedicated notifications page. Use JavaScript to update the UI in real-time when new notifications arrive via WebSocket.
- Notification Settings: Allow users to customize their notification preferences, such as turning notifications on/off for specific events or channels.
- Testing and Debugging: Thoroughly test your real-time notification system to ensure it works as expected. Debug any issues with WebSocket connections or message delivery.
- Scalability: As your application grows, consider how to scale your real-time notification system to handle a larger number of concurrent users. You may need to deploy additional WebSocket servers or use technologies like Redis for scaling.
- Security: Ensure that your real-time communication is secure. Use secure WebSocket connections (wss://), and implement authentication and authorization mechanisms to prevent unauthorized access to notifications.
Implementing real-time notifications in Django requires a combination of back-end and front-end development skills. It can significantly improve user engagement and provide a more dynamic user experience. Make sure to keep your code well-organized and maintainable as your notification system evolves with your application.
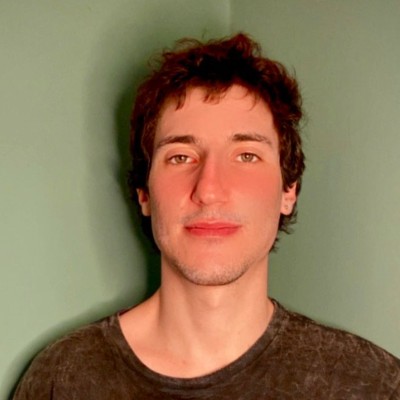
Previously at
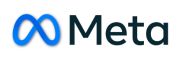
Experienced Full-stack Developer with a focus on Django, having 7 years of expertise. Worked on diverse projects, utilizing React, Python, Django, and more.