How to implement token-based authentication in Django REST framework?
Implementing token-based authentication in Django REST framework (DRF) is a common and effective way to secure your API by requiring users to present a unique token with each request. Here’s a step-by-step guide to implementing token-based authentication in DRF:
- Install DRF and Authentication Libraries:
First, ensure you have DRF installed in your Django project. You’ll also need a library for token-based authentication, such as `djangorestframework.authtoken`. You can install it using pip:
```bash pip install djangorestframework pip install djangorestframework-authtoken ```
- Configure Authentication:
In your project’s settings, add `’rest_framework.authtoken’` to the `INSTALLED_APPS` list and configure DRF to use token authentication:
```python INSTALLED_APPS = [ # ... 'rest_framework', 'rest_framework.authtoken', # ... ] REST_FRAMEWORK = { 'DEFAULT_AUTHENTICATION_CLASSES': ( 'rest_framework.authentication.TokenAuthentication', ), } ```
- Create Tokens:
Run Django’s migration commands to create the necessary database tables for tokens:
```bash python manage.py makemigrations python manage.py migrate ```
- Obtain Tokens:
When a user registers or logs in, generate a token for them. You can create a view or endpoint to issue tokens. Here’s an example using DRF’s built-in views:
```python from rest_framework.authtoken.views import ObtainAuthToken urlpatterns = [ # ... path('api-token-auth/', ObtainAuthToken.as_view(), name='api_token_auth'), # ... ] ```
Clients can make a POST request to this endpoint with their username and password to obtain a token.
- Include Tokens in Requests:
Clients must include their token in the `Authorization` header of each request. The header should be in the format: `Authorization: Token <token_key>`.
- Protect Views:
To secure views or viewsets, apply the `IsAuthenticated` permission class to require token-based authentication. For example:
```python from rest_framework.permissions import IsAuthenticated class MyView(APIView): permission_classes = [IsAuthenticated] # Your view logic here ```
By following these steps, you can implement token-based authentication in your Django REST framework API. This approach enhances security by ensuring that only authenticated users with valid tokens can access protected resources. It’s a robust solution for securing your API endpoints and protecting sensitive data.
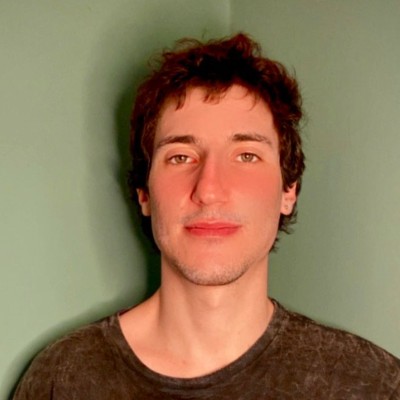
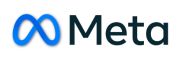