How to implement two-factor authentication (2FA) in Django?
Implementing two-factor authentication (2FA) in Django adds an extra layer of security to user accounts by requiring users to provide two different authentication factors: something they know (password) and something they have (a one-time code from a mobile app or SMS). Here’s a step-by-step guide on how to implement 2FA in Django:
- Choose a 2FA Method:
Decide which 2FA method you want to implement, such as Time-based One-Time Passwords (TOTP) or SMS-based authentication. TOTP is recommended for its security and ease of use.
- Install Required Packages:
Depending on your chosen method, install the necessary Django packages. For TOTP, you can use packages like `django-otp` and `django-otp-plugins`.
- Enable 2FA for Users:
Create a model or extend Django’s built-in User model to store user 2FA settings. Add fields like `totp_secret_key` and `is_2fa_enabled`.
- Generate and Store TOTP Secrets:
When a user enables 2FA, generate a TOTP secret key for them and store it securely in the database. You can use the `pyotp` library to generate TOTP codes.
- Create 2FA Views and Templates:
Build views and templates for users to enable, disable, and manage 2FA settings. Allow users to scan a QR code to set up their 2FA app (like Google Authenticator).
- Verify TOTP Codes:
Implement a view to verify TOTP codes provided by users during login. Use the `pyotp` library to verify the codes against the stored secret keys.
- Fallback Mechanism:
Implement a fallback mechanism for users who can’t use TOTP, such as SMS-based codes or backup codes. Ensure you have secure mechanisms for sending and storing backup codes.
- Security Measures:
Implement security measures like rate limiting for code verification attempts to prevent brute-force attacks.
- Testing:
Thoroughly test your 2FA implementation to ensure it works correctly and securely.
- User Education:
Educate your users about 2FA and how to set it up. Provide clear instructions and support.
- Logging and Monitoring:
Implement logging for 2FA-related activities and set up monitoring to detect any unusual 2FA-related events.
- Compliance and Regulations:
Ensure your 2FA implementation complies with relevant regulations, especially if your application handles sensitive data.
- Continuous Maintenance:
Regularly review and update your 2FA implementation to address security concerns and adapt to new threats.
By following these steps, you can successfully implement two-factor authentication (2FA) in your Django application, enhancing security for your users’ accounts and data. Remember to prioritize user experience and make the setup process as user-friendly as possible to encourage adoption.
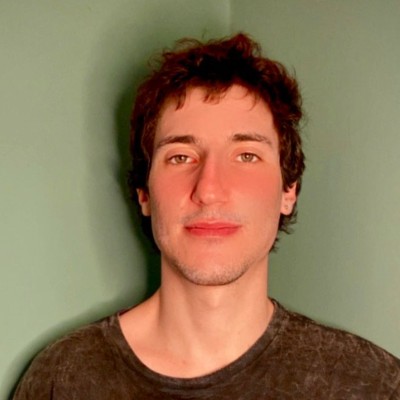
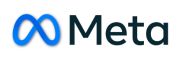