Django Q & A
How to implement user profiles in Django?
Implementing user profiles in Django is a common requirement for extending user data beyond the built-in authentication system. User profiles allow you to store additional information about users, such as their profile picture, bio, and preferences. Here’s a step-by-step guide on how to implement user profiles in Django:
- Create a Profile Model: Start by creating a user profile model that extends Django’s built-in `User` model or any custom user model you may be using. This model will store the additional user information. For example:
```python from django.db import models from django.contrib.auth.models import User class UserProfile(models.Model): user = models.OneToOneField(User, on_delete=models.CASCADE) profile_picture = models.ImageField(upload_to='profile_pics/', null=True, blank=True) bio = models.TextField(max_length=500, null=True, blank=True) # Add other fields as needed ```
- Create a Profile Form: Build a form for users to update their profile information. You can use Django’s `ModelForm` to create a form automatically based on your profile model.
- User Registration and Profile Creation: When a user registers, create a user profile instance associated with the user. This can be done in your registration view or by using signals like `post_save` to automatically create a profile when a new user is saved.
- Profile Editing: Implement views and templates to allow users to edit their profiles. These views should handle form submission and update the user profile accordingly.
- Profile Display: Create a user profile view that displays the user’s profile information to others. Customize the template to present the information in a user-friendly way.
- User Authentication: Ensure that only authenticated users can access profile editing and display views by using Django’s authentication decorators or middleware.
- Permissions: Define permissions to control who can edit or view user profiles. You can use Django’s built-in permission system or implement custom logic as needed.
- Security: Implement security measures like proper form validation, user input sanitation, and image upload validation to prevent security vulnerabilities.
By following these steps, you can effectively implement user profiles in Django, providing users with the ability to personalize their information and enhance their engagement with your application. User profiles are a valuable feature for creating a more interactive and user-centered web experience.
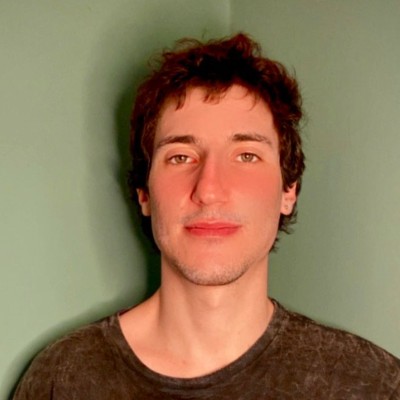
Previously at
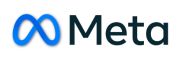
Experienced Full-stack Developer with a focus on Django, having 7 years of expertise. Worked on diverse projects, utilizing React, Python, Django, and more.