How to implement WebSockets using Django channels?
Implementing WebSockets in Django using Django Channels allows you to build real-time and interactive web applications. WebSockets provide full-duplex communication channels over a single TCP connection, enabling server-to-client and client-to-server communication without the need for constant HTTP requests. Here’s how you can implement WebSockets in Django using Django Channels:
- Install Django Channels:
Begin by installing Django Channels using pip:
```bash pip install channels ```
- Configure Django Settings:
In your Django project’s settings (`settings.py`), add Channels to your `INSTALLED_APPS` and configure the channel layer backend. You can use Redis or other backends for this purpose. For Redis, you can install it and configure it in settings:
```python # settings.py INSTALLED_APPS = [ # ... 'channels', ] # Configure the channel layer CHANNEL_LAYERS = { "default": { "BACKEND": "channels_redis.core.RedisChannelLayer", "CONFIG": { "hosts": [("localhost", 6379)], }, }, } ```
- Create a Consumer:
Consumers are similar to Django views but are designed for handling WebSocket connections. Create a consumer by subclassing `AsyncWebsocketConsumer` and defining methods to handle different WebSocket events.
```python # consumers.py from channels.generic.websocket import AsyncWebsocketConsumer import json class MyConsumer(AsyncWebsocketConsumer): async def connect(self): await self.accept() async def disconnect(self, close_code): pass async def receive(self, text_data): data = json.loads(text_data) message = data['message'] await self.send(text_data=json.dumps({ 'message': message })) ```
- Routing Configuration:
Configure routing to connect WebSocket requests to your consumer. Create a `routing.py` file to define routing rules:
```python # routing.py from django.urls import re_path from . import consumers websocket_urlpatterns = [ re_path(r'ws/some_path/$', consumers.MyConsumer.as_asgi()), ] ```
- Include Routing in Your Django Application:
In your project’s `asgi.py` or `wsgi.py` file, include the WebSocket routing:
```python # asgi.py import os from django.core.asgi import get_asgi_application from channels.routing import ProtocolTypeRouter, URLRouter os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'your_project.settings') application = ProtocolTypeRouter({ "http": get_asgi_application(), "websocket": URLRouter( # Add your WebSocket routing here routing.websocket_urlpatterns ), }) ```
- JavaScript on the Client Side:
On the client side, use JavaScript to establish and manage WebSocket connections. You can use libraries like `WebSocket` or higher-level libraries like `Socket.io` for this purpose.
With these steps, you can implement WebSockets in Django using Django Channels. This setup enables real-time communication between clients and the server, making it suitable for chat applications, notifications, live updates, and more.
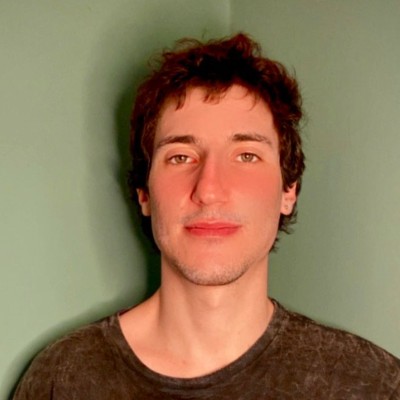
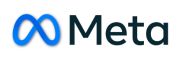