How In-Memory Caching Can Transform Your Django Application
In the world of web development, a crucial aspect that often requires attention is improving performance. A frequently employed technique to boost performance is “caching.” Caching is a method of storing the result of an expensive computation so that it can be quickly retrieved in the future without needing to be recomputed. Django, a popular Python web framework, provides several ways to use caching effectively. That’s why many firms are looking to hire Django developers who are proficient in these techniques.
Table of Contents
This article will delve into the concept of caching, its role in Django applications, and, specifically, how we can leverage in-memory caching using systems like Memcached or Redis to optimize your Django web applications’ performance. So, whether you’re a seasoned Django developer or an organization looking to hire Django developers, this in-depth exploration of caching in Django will be beneficial. Let’s dive right in!
1. The Basics of Caching
In simple terms, caching is storing the result of a particular operation for future use. Let’s consider a hypothetical scenario: Suppose you’re making a complex database query to fetch user details based on a set of conditions. The first time you make the query, the database needs to work hard to gather all the details you need. But what if you could save the result somewhere, and the next time you need that information, instead of querying the database again, you can just retrieve the already stored data? That’s precisely what caching does!
2. Caching in Django
Django offers a robust and versatile cache framework that lets you save your dynamic pages so they don’t have to be processed for each request. You can choose to cache the output of specific views, the output of certain template fragments, or even an entire site.
Caching in Django can be achieved at several levels:
- View Level: Here, the cache framework stores the entire result of a view function for a given set of input parameters.
- Template Level: This level of caching enables storing the output of certain portions of your templates (template fragments) that may be expensive to calculate.
- Low-Level (Manual) Caching: This level allows you to cache any Python data structure, but you need to handle the cache keys (the names that you give to your cached items) manually.
- Middleware Level: This level allows you to cache your entire website.
3. In-Memory Caching: Memcached and Redis
In-memory caching is a technique where the cache data is stored in the main memory (RAM) of your server, which is considerably faster to access than typical disk storage. In-memory cache systems like Memcached or Redis are commonly used due to their efficiency and speed.
3.1 Memcached
Memcached is a high-performance, distributed memory object caching system. It allows you to take memory from parts of your system where you have more than you need and use it where you need more.
To utilize Memcached in Django, you need to install the `python-memcached` module and modify your settings.py file as follows:
```python CACHES = { 'default': { 'BACKEND': 'django.core.cache.backends.memcached.PyMemcacheCache', 'LOCATION': '127.0.0.1:11211', } } ```
Here, `LOCATION` is where your Memcached server is running.
3.2 Redis
Redis is an open-source, in-memory data structure store that is used as a database, cache, and message broker. It supports various data structures and offers replication and different levels of on-disk persistence.
To utilize Redis as your cache backend in Django, install the `django-redis` module and update your settings.py file:
```python CACHES = { 'default': { 'BACKEND': 'django_redis.cache.RedisCache', 'LOCATION': 'redis://127.0.0.1:6379/1', 'OPTIONS': { 'CLIENT_CLASS': 'django_redis.client.DefaultClient', } } } ```
Here, `LOCATION` is your Redis server’s address.
4. Practical Example: Boosting Performance with In-Memory Caching
Now, let’s take a look at a practical example where we can boost a Django application’s performance with in-memory caching. Suppose we have an eCommerce website where we frequently need to display the top 10 selling products.
```python def top_products(request): products = Product.objects.order_by('-sales')[:10] return render(request, 'top_products.html', {'products': products}) ```
Here, each time a request is made to view the top 10 products, Django hits the database. However, we can improve this with caching.
```python from django.core.cache import cache def top_products(request): products = cache.get('top_products') if not products: products = Product.objects.order_by('-sales')[:10] cache.set('top_products', products, 3600) # Cache data for one hour. return render(request, 'top_products.html', {'products': products}) ```
In this updated version, we first try to get the ‘top_products’ from the cache. If they’re not in the cache (i.e., `cache.get(‘top_products’)` returns `None`), we hit the database to fetch the data, and then we cache this data for one hour. So, for the next hour, any request to see the top 10 products will be served from the cache, reducing the load on your database and increasing the speed of the request.
Conclusion
Caching is a potent tool in web development, especially for high-traffic sites. Django’s cache framework, coupled with in-memory cache systems like Memcached or Redis, offers an excellent solution for boosting your application’s performance. While caching can significantly improve speed, it’s essential to remember that it adds another layer of complexity to your system. The correct caching strategies depend on your application’s specific requirements, so a thoughtful balance between speed and accuracy of data must be maintained. Remember, caching should not be used as a band-aid solution to mask inefficient queries or poor database design.
In-memory caching in Django is an exciting field and a critical skill for developers to master, which is why many organizations choose to hire Django developers with this expertise. Whether you’re working on a personal project, hiring Django developers for your team, or building large-scale applications, knowledge of in-memory caching will undoubtedly help improve the efficiency and speed of your Django applications.
Table of Contents
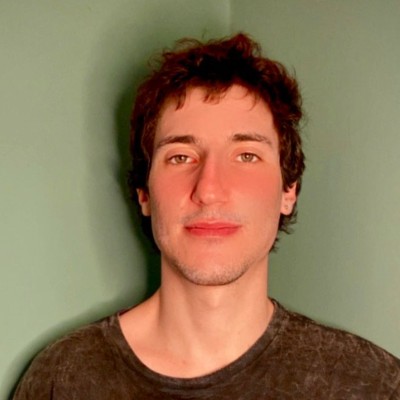
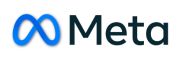