Django Developer Interview Questions Guide: Find Your Ideal Candidate
Django is a popular Python framework for building web applications. If you’re looking to hire Django developers, it’s crucial to assess their technical skills, problem-solving abilities, and experience with Django and its ecosystem. This is where Django developer interview questions play a vital role.
Table of Contents
In this article, we will discuss some of the most important interview questions to ask a Django developer in order to find the perfect fit for your team.
1. How to Hire Django Developers
When hiring Django developers, there are various methods you can use, such as job postings, online platforms, and recruiters. The first step is to clearly define the job requirements and the skills and experience you are looking for in a candidate.
Top Skills of Django Developers to Look Out For:
In addition to Django expertise, here are some key skills to consider when hiring Django developers:
- Proficiency in Python: A strong understanding of the Python programming language is essential.
- Django framework knowledge: Look for candidates who have experience building and maintaining web applications using Django.
- Database skills: Evaluate their understanding of working with databases, SQL, and Django’s ORM for data modeling and querying.
- Front-end skills: Assess their familiarity with front-end technologies like HTML, CSS, and JavaScript, as Django developers often work on the presentation layer.
- Testing and debugging: Look for experience with testing frameworks like pytest or Django’s built-in testing tools, as well as their ability to debug and troubleshoot issues.
- Version control systems: Familiarity with Git and other version control systems is important for collaboration.
2. Overview of the Django Developer Hiring Process
To interview a Django developer effectively, it’s important to understand their technical knowledge, problem-solving abilities, and overall fit for the role. Here’s an overview of the hiring process:
2.1 Defining the Job Requirements and Required Skills
Clearly define the job requirements and the specific skills and experience you are seeking in a Django developer. This includes technical skills such as proficiency in Django, Python, and databases, as well as soft skills like communication and teamwork.
2.2 Creating an Effective Job Description
Craft a compelling job description that accurately reflects the role of a Django developer within your organization. Use a clear job title, such as Django Developer or Python Web Developer, to attract the right candidates.
2.3 Preparing Django Developer Interview Questions
Prepare a set of technical questions to assess the candidate’s understanding of Django concepts, web development, database management, and problem-solving abilities. Consider including coding challenges or real-world scenarios to gauge their practical knowledge.
Here are some sample Django developer interview questions and possible answers you may want to include in your assessment:
Q1. Can you explain the MVC architectural pattern and how it relates to Django?
MVC stands for Model-View-Controller. In Django, the model represents the data and database schema, the view handles the presentation logic, and the controller manages the interaction between the model and the view. Django follows a similar architectural pattern, where models represent database tables, views handle the request/response logic, and templates handle the presentation.
Q2. How do you define models and create database tables in Django?
In Django, models are defined as Python classes that inherit from the django.db.models.Model
base class. Each class attribute represents a database field. To create database tables from the defined models, you need to run migrations using the python manage.py makemigrations
and python manage.py migrate
commands.
Q3. Can you explain the purpose of Django’s ORM (Object-Relational Mapping)?
Django’s ORM allows developers to interact with databases using Python code instead of writing raw SQL queries. It provides a high-level API for creating, retrieving, updating, and deleting database records. The ORM handles the mapping between the database tables and Python objects.
Q4. How do you handle form validation and processing in Django?
Django provides a form handling framework that simplifies form validation and processing. Developers define form classes using Django’s forms.Form
or forms.ModelForm
classes. The framework handles validating user input, displaying errors, and processing the form data.
Q5. Can you explain the concept of Django’s URL routing and provide an example?
URL routing in Django maps URLs to views. Developers define URL patterns using regular expressions or path patterns in the urls.py
file. Each URL pattern is associated with a view function or class-based view. For example, a URL pattern /articles/<int:id>/
can be mapped to a view function that retrieves and displays an article based on the provided ID.
Q6. How do you use Django’s built-in authentication system?
Django provides a robust authentication system out of the box. It includes features like user registration, login, logout, password management, and user permissions. Developers can use Django’s authentication views, forms, and middleware to handle user authentication and authorization.
Q7. What are Django’s class-based views and how are they useful?
Django’s class-based views (CBVs) are an alternative to function-based views. CBVs are reusable classes that encapsulate the logic for handling specific HTTP requests. They provide a structured approach to defining views, promoting code reusability and reducing code duplication.
Q8. Can you explain the concept of middleware in Django?
Middleware in Django is a component that sits between the web server and the view. It processes requests and responses, allowing developers to modify or analyze them. Middleware can perform tasks such as authentication, session management, or adding custom headers.
Q9. How do you work with static files and media files in Django?
Django provides built-in support for managing static files (e.g., CSS, JavaScript) and media files (e.g., user uploads). Static files are typically served by the web server directly, while media files are stored on the server’s file system. Developers configure the file storage settings in Django’s settings file.
Q10. Can you explain the purpose of Django’s template engine and provide an example template?
Django’s template engine allows developers to separate the presentation logic from the application logic. Templates are HTML files with embedded template tags and variables. They can contain loops, conditionals, and filters. An example template code might look like:
<h1>{{ article.title }}</h1> <p>{{ article.content }}</p>
Q11. How do you optimize database queries in Django?
Optimizing database queries in Django involves using techniques like selecting only necessary fields, using select_related
and prefetch_related
to minimize database round-trips, and leveraging caching mechanisms. Developers can also use Django’s query optimization tools like select_related
, prefetch_related
, and query profiling tools.
Q12. Can you explain the concept of migrations in Django?
Migrations in Django are a way to manage database schema changes over time. They allow developers to update the database structure without losing data. Migrations are defined as Python files that contain operations to apply or revert changes to the database schema.
Q13. How do you handle caching in Django applications?
Django provides built-in caching mechanisms to improve performance. Developers can cache the results of expensive queries, rendered templates, or parts of a view using decorators or middleware. Django supports various caching backends like in-memory caching or external caches like Memcached or Redis.
Q14. What is Django REST framework and how is it used for building APIs?
Django REST framework is a powerful toolkit for building Web APIs. It provides a set of classes and functionalities to simplify API development, including serialization, authentication, permissions, and viewsets. Django REST framework allows developers to quickly build robust and scalable APIs.
Q15. Can you explain the concept of Django’s signals and provide an example use case?
Django’s signals allow decoupled applications to get notified when certain actions occur. Signals provide a way to allow communication between different parts of an application without tight coupling. An example use case is triggering an email notification when a new user registers.
Q16. How do you handle internationalization and localization in Django?
Django provides extensive support for internationalization (i18n) and localization (l10n). Developers can mark translatable strings in their code using Django’s translation functions and then generate language-specific versions of the text. Django handles language switching and provides tools for translating content.
Q17. Have you worked with any testing frameworks in Django?
Testing is an essential part of Django development. Look for candidates familiar with testing frameworks like Django’s built-in unittest
module or third-party libraries like pytest. Candidates should demonstrate knowledge of writing unit tests, integration tests, and using testing utilities provided by Django.
Q18. Write a Django view function that takes an HTTP POST request with JSON data and saves it to a database model called Book
.
The JSON data should include fields for title
, author
, and published_date
. Example answer:
from django.http import JsonResponse from django.views.decorators.csrf import csrf_exempt import json from .models import Book @csrf_exempt def save_book(request): if request.method == 'POST': data = json.loads(request.body) title = data.get('title') author = data.get('author') published_date = data.get('published_date') book = Book(title=title, author=author, published_date=published_date) book.save() return JsonResponse({'message': 'Book saved successfully'}) else: return JsonResponse({'message': 'Invalid request'})
Q19. Implement a Django template filter called truncate_chars
that takes a string as input and truncates it to a specified number of characters, adding ellipsis at the end if necessary.
Example answer:
from django import template register = template.Library() @register.filter def truncate_chars(value, length): if len(value) <= length: return value else: return value[:length] + '...'
Usage in a template: {{ my_text|truncate_chars:50 }}
Q20. Write a Django model method called get_absolute_url
that returns the URL for accessing a specific instance of the model. Assume the model has a unique id
field.
Example answer:
from django.urls import reverse from django.db import models class MyModel(models.Model): # fields and methods def get_absolute_url(self): return reverse('mymodel-detail', kwargs={'pk': self.id})
In this example, replace 'mymodel-detail'
with the appropriate URL pattern name for accessing the specific model instance.
These questions and code samples will help you assess a candidate’s ability to write Django code and solve problems using the framework. The provided answers showcase the expected implementation, but candidates may provide alternative solutions that achieve the same goal.
3. Where Can You Find the Right Django Dev Candidates to Interview?
To find the right Django developer candidates, you can use various methods. Post job advertisements on platforms like LinkedIn, Indeed, or specialized tech job boards. Additionally, consider leveraging online platforms like freelancer websites or remote work marketplaces that specifically cater to Django developers.
For a streamlined hiring process that saves time and effort, consider using a developer platform like CloudDevs. CloudDevs offers a pool of pre-screened senior Django developers, eliminating the need for extensive interview and screening processes.
4. How to Hire Django Devs through CloudDevs?
CloudDevs simplifies the process of hiring Django developers in three steps:
Step 1: Connect with CloudDevs: Schedule a call with a CloudDevs consultant to discuss your project requirements, scope, desired skills, and expected experience.
Step 2: Find a Talent Match: Within 24 hours, CloudDevs presents you with shortlisted candidates from their pool of pre-vetted senior Django developers. Review their profiles and choose the candidate that best fits your project.
Step 3: Start Your No-Risk Trial: Get on a call with your selected Django developer to familiarize yourself. Once satisfied, sign the contract and begin your project with a week-long free trial.
By utilizing CloudDevs’ platform, you can find and hire top Django developers effortlessly, ensuring your team possesses the necessary skills and expertise to build exceptional web applications.
In conclusion, by following these steps and asking the provided Django developer interview questions, you can effectively evaluate candidates’ coding skills, problem-solving abilities, and overall fit for your organization. Whether you choose traditional methods or utilize a developer platform like CloudDevs, these interview questions will help you identify the perfect Django developer for your team.
Table of Contents
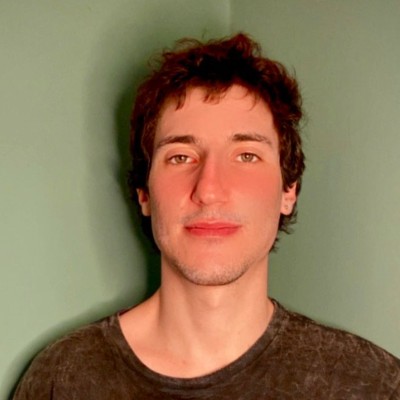
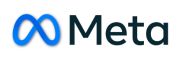