Why Django is the Perfect Framework for Your IoT Projects
The Internet of Things (IoT) is a revolutionary concept that continues to shape our world in unprecedented ways, by enabling physical objects to connect and communicate over the internet. As IoT becomes increasingly mainstream, many developers are leveraging popular frameworks like Django to build robust IoT applications. Django, a high-level Python web framework, is lauded for its simplicity, flexibility, and scalability – qualities that make it an excellent choice for IoT development.
Table of Contents
This blog post is a comprehensive exploration of how to leverage Django in the context of IoT. We will look at why Django is suited for IoT applications and walk through examples of how to build a Django-powered IoT system.
1. Why Django for IoT?
- Python Compatibility: Python is the leading language in IoT development due to its simplicity and readability, along with a rich set of libraries and frameworks that facilitate IoT solutions. Django, being a Python-based framework, benefits from all these perks, thus being an excellent choice for IoT development.
- Scalability: Django is designed to help developers take applications from concept to completion as quickly as possible. It can handle heavy traffic demands of IoT systems with its ability to scale quickly and flexibly.
- Security: Django places a high emphasis on security, helping developers avoid common security mistakes such as cross-site scripting, cross-site request forgery, and SQL injection. This is particularly important in IoT where data security is paramount.
- Maturity: Django has been around for a while, meaning there’s a wealth of documentation, tutorials, and community support available. This can dramatically speed up the development process and offer solutions to common problems encountered.
2. Building a Django-powered IoT system
We’ll now explore how to use Django to build a simple IoT system that collects temperature and humidity data from a sensor, stores it in a database, and displays it on a web interface. This example is based on a Raspberry Pi device, but the concepts can be applied to any IoT device.
*Disclaimer: This example assumes you have a working knowledge of Python and Django, as well as basic knowledge of IoT hardware.*
Step 1: Setting up the Hardware
We’ll need a DHT11 temperature and humidity sensor, a Raspberry Pi, and jumper wires for our setup. Connect the DHT11 sensor to your Raspberry Pi, following appropriate tutorials online.
Step 2: Installing Django and Dependencies
On your Raspberry Pi, install Django along with other necessary dependencies:
``` pip install Django pip install Adafruit_DHT ```
Step 3: Creating the Django Project and App
Create a new Django project:
``` django-admin startproject weather_station ```
Then, navigate to your new project and create a new app:
``` cd weather_station django-admin startapp sensor_data ```
Step 4: Setting up the Models
In `sensor_data/models.py`, define your data model:
```python from django.db import models class SensorData(models.Model): temperature = models.FloatField() humidity = models.FloatField() created_at = models.DateTimeField(auto_now_add=True) ```
Then, add `sensor_data` to your installed apps in `weather_station/settings.py`:
```python INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'sensor_data', ] ```
Run the migration command to create the database schema:
``` python manage.py makemigrations sensor_data python manage.py migrate ```
Step 5: Reading Sensor Data
Create a new Python file `read_sensor.py` in your app directory:
```python import Adafruit_DHT from sensor_data.models import SensorData def read_and_store_sensor_data(): humidity, temperature = Adafruit_DHT.read_retry(Adafruit_DHT.DHT11, 4) if humidity is not None and temperature is not None: SensorData.objects.create(temperature=temperature, humidity=humidity) else: print('Failed to get reading. Try again!') ```
This function reads data from the sensor and stores it in the database.
Step 6: Displaying Data in a Web Interface
In your `sensor_data/views.py`:
```python from django.shortcuts import render from .models import SensorData def sensor_data_view(request): sensor_data = SensorData.objects.all().order_by('-created_at') return render(request, 'sensor_data.html', {'sensor_data': sensor_data}) ```
Then, create a new template `sensor_data.html` in a templates directory within your app directory:
```html <!DOCTYPE html> <html> <head> <title>Weather Station</title> </head> <body> <h1>Temperature and Humidity Data</h1> <table> <tr> <th>Time</th> <th>Temperature</th> <th>Humidity</th> </tr> {% for data in sensor_data %} <tr> <td>{{ data.created_at }}</td> <td>{{ data.temperature }}</td> <td>{{ data.humidity }}</td> </tr> {% endfor %} </table> </body> </html> ```
Add a URL for this view in your `weather_station/urls.py`:
```python from django.urls import path from sensor_data.views import sensor_data_view urlpatterns = [ path('sensor_data/', sensor_data_view), ] ```
Step 7: Running the Server
You can now start the Django development server:
``` python manage.py runserver 0.0.0.0:8000 ```
You should be able to access the web interface by navigating to `<Your Raspberry Pi’s IP Address>:8000/sensor_data/` in a web browser.
Conclusion
This simple example only scratches the surface of what’s possible with Django in the context of IoT. The scalability and flexibility of Django, combined with the vast capabilities of Python, open up a world of possibilities for building powerful, robust IoT applications. Whether it’s home automation systems, industrial IoT, or smart city applications, Django can be an excellent choice for your IoT development needs.
Table of Contents
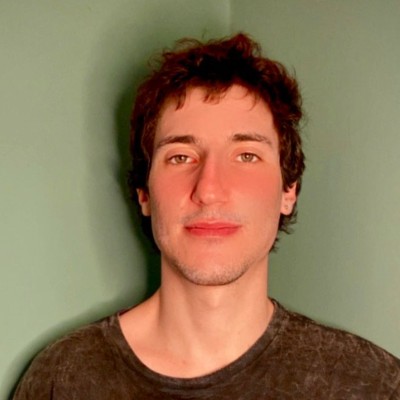
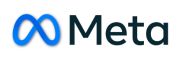