From IoT Devices to Web: Using Django for Seamless Data Integration
The Internet of Things (IoT) has opened up new horizons for numerous industries by offering a connected ecosystem of devices. From smart homes to industrial automation, IoT sensors play a pivotal role in data collection and communication. Django, a robust and versatile web framework, is a great tool for collecting, storing, and analyzing this sensor data. In this post, we’ll walk you through the process of setting up a Django application that interfaces with IoT sensors.
Table of Contents
1. The Basics: What Are IoT Sensors?
IoT sensors are typically small hardware devices that collect information from their environment. This data can be anything from temperature, humidity, light intensity, or even motion. The data is then transmitted to other devices, cloud platforms, or local servers for further processing or action.
2. Setting Up Django for IoT
Before we begin, ensure you have Django installed. If not, install it using pip:
```bash pip install django ```
- Create a new Django project:
```bash django-admin startproject iot_project ```
- Create an app called ‘sensor_data’:
```bash cd iot_project python manage.py startapp sensor_data ```
Add `sensor_data` to `INSTALLED_APPS` in `settings.py`:
```python INSTALLED_APPS = [ ..., 'sensor_data', ] ```
3. Designing the Database Model
For this example, we’ll be working with a temperature sensor.
3.1. Update `models.py` in `sensor_data` app:
```python from django.db import models class TemperatureSensor(models.Model): timestamp = models.DateTimeField(auto_now_add=True) temperature = models.FloatField() def __str__(self): return f"{self.temperature}°C at {self.timestamp}" ```
3.2. Migrate the database:
```bash python manage.py makemigrations python manage.py migrate ```
4. Data Collection Endpoint
Django’s ability to set up HTTP endpoints makes it a perfect choice to interface with IoT devices.
4.1. Set up a URL route in `urls.py` of the `sensor_data` app:
```python from django.urls import path from . import views urlpatterns = [ path('collect/', views.collect_data, name="collect_data"), ] ```
4.2. Create the `collect_data` view in `views.py`:
```python from django.http import JsonResponse from .models import TemperatureSensor def collect_data(request): if request.method == 'GET': temperature = request.GET.get('temperature', None) if temperature: TemperatureSensor.objects.create(temperature=float(temperature)) return JsonResponse({"status": "success"}) else: return JsonResponse({"status": "error", "message": "Temperature data missing."}) ```
Any IoT sensor device can send a GET request to this endpoint with temperature data. For example: `http://yourserver.com/collect/?temperature=25.6`.
5. Analyzing Sensor Data
With data in our database, we can implement visualization.
5.1. Create a view to display temperature data:
In `views.py`:
```python from django.shortcuts import render def display_data(request): data = TemperatureSensor.objects.all() return render(request, 'data_display.html', {'data': data}) ```
5.2. Create a template `data_display.html` in `sensor_data/templates`:
```html <!DOCTYPE html> <html> <head> <title>Temperature Data</title> </head> <body> <h1>Temperature Readings</h1> <ul> {% for reading in data %} <li>{{ reading }}</li> {% endfor %} </ul> </body> </html> ```
5.3. Link to the `display_data` view in `urls.py`:
```python urlpatterns = [ ..., path('display/', views.display_data, name="display_data"), ] ```
Visiting `http://yourserver.com/display/` will show a list of temperature readings.
Conclusion
Django’s versatility and ease-of-use make it a prime choice for interfacing with IoT sensors. This guide showcases a basic setup to collect and display data from temperature sensors, but the principles can be extended for more complex sensors and use-cases. With Django, the sky’s the limit when it comes to IoT data collection, storage, and analysis!
Table of Contents
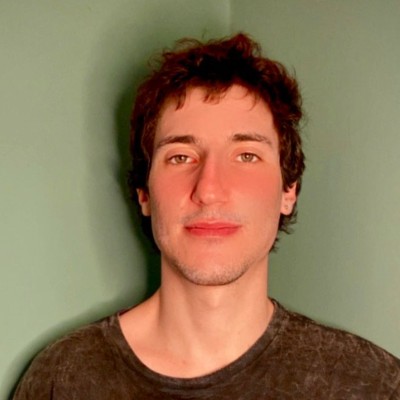
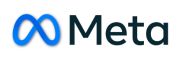