Explore the Intersection of Django and Machine Learning in Web Development
Machine Learning (ML) has been at the forefront of technological advancement in recent years, leading to innovative solutions across numerous industries. Django, a high-level Python web framework, has become a popular choice for web developers due to its simplicity and scalability. Combining these two technologies can create powerful, intelligent web applications that leverage machine learning capabilities. This blog post will explore how to incorporate machine learning models into your Django web application using practical examples.
Table of Contents
1. Introduction to Django and Machine Learning
Django, built on Python, makes it possible for developers to build robust, scalable, and maintainable web applications rapidly. It’s a perfect choice for handling complex, data-driven websites, and it’s equipped with a rich ecosystem of tools and libraries.
Machine Learning, on the other hand, is a subset of artificial intelligence (AI) that provides systems with the ability to learn from data and improve performance over time. Python, being the most popular language in the ML community, comes with a wide array of libraries like Scikit-learn, TensorFlow, PyTorch, etc., to develop and deploy machine learning models.
Integrating a machine learning model into a Django web app can open up new possibilities. You could build a web app that classifies images, predicts trends, or even personalizes user experiences.
2. How to Incorporate ML Models in Django
Step 1: Building/Choosing the Machine Learning Model
Before you can integrate a machine learning model into Django, you need to have a model. You can either use pre-trained models or build your own using ML libraries.
For instance, let’s assume we’re building a web app that uses a predictive model to estimate house prices. We can use Scikit-learn, a popular ML library, to create our model. Below is a simplified example:
```python from sklearn.datasets import load_boston from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression # Load the dataset data = load_boston() # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(data.data, data.target, test_size=0.2) # Initialize the model model = LinearRegression() # Train the model model.fit(X_train, y_train) # You can now use model.predict() to predict house prices ```
After creating the model and testing its accuracy, you need to save it using a tool like joblib or pickle so that it can be reloaded in your Django app:
```python import joblib # Save the model joblib.dump(model, 'house_price_model.pkl') ```
Step 2: Setting Up Your Django Web App
Once you have your machine learning model, it’s time to create your Django web application. After installing Django, you can create a new project using the command `django-admin startproject house_price_prediction`. This will create a new Django project.
Next, create an app within the project using the command `python manage.py startapp prediction`. This will create a new Django app called “prediction.”
Step 3: Integrating the ML Model into Django
You can integrate the ML model by loading it into your Django view, where you can use it to make predictions based on user input. Below is an example of how to achieve this:
First, make sure you place your `house_price_model.pkl` file in a location that’s accessible to the Django app. A good place could be inside the `prediction` app directory.
Here is a simplified view that loads the model and uses it to make a prediction:
```python from django.http import JsonResponse from sklearn.externals import joblib def predict_price(request): # Load the model model = joblib.load("prediction/house_price_model.pkl") # Assume that "features" are sent as GET parameters features = [float(request.GET.get(f, 0)) for f in ('average_rooms', 'crime_rate', 'tax_rate', etc.)] # Make the prediction prediction = model.predict([features]) # Return the prediction as a JSON response return JsonResponse({'prediction': prediction[0]}) ```
In this case, the Django view expects the features for the prediction to be provided as GET parameters in the request. The features are used to make a prediction, which is returned as a JSON response.
Step 4: Creating a User Interface
Once you have integrated the ML model into Django, the final step is to create a user interface where users can enter data and see the predictions.
For this, you would need to create a Django form and a template. Users will fill out the form with the required data, which will then be sent to the server. The server will make a prediction based on this data and return the result, which can then be displayed on the website.
The form might look something like this:
```python from django import forms class HousePricePredictionForm(forms.Form): average_rooms = forms.FloatField() crime_rate = forms.FloatField() tax_rate = forms.FloatField() # and so on for each feature... ```
And you can create a template to display this form to the user, collect the data, and display the predicted price.
Conclusion
Integrating machine learning models with web applications adds an extra layer of functionality and interactivity, offering users real-time insights and predictions. Django, with its Pythonic nature, is an excellent framework to deploy such models due to the vast machine learning libraries available in Python.
Keep in mind, this post illustrates a very simplified approach to integrating ML models into a Django app. Depending on your use case, you might need to consider data pre-processing, ensuring the model’s accuracy, or even implementing real-time model training within your app. Regardless, this guide provides you a solid starting point for integrating ML models into Django.
Table of Contents
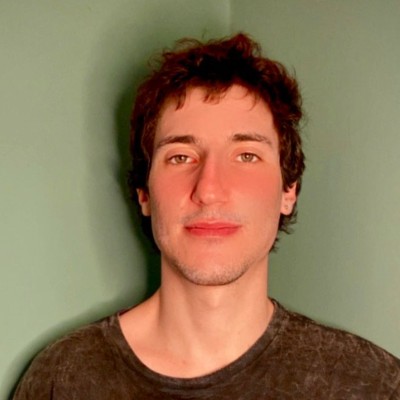
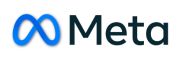