What are Django Mixins, and how to use them?
Django mixins are a powerful tool in Django for code reuse and building flexible class-based views. They allow you to encapsulate and share common functionality among multiple views without the need for inheritance, promoting cleaner and more maintainable code.
Here’s how Django mixins work and how to use them:
Understanding Mixins:
Mixins are essentially small, reusable classes that contain specific methods or attributes. They are not meant to be used on their own but rather mixed into your view classes. Mixins provide a way to modularize your code, making it easier to manage and extend.
Creating Mixins:
To create a mixin, you define a class with the methods or attributes you want to reuse across different views. For example, you might create an `AuthMixin` that includes authentication checks:
```python class AuthMixin: def check_authentication(self): # Your authentication logic here ```
Using Mixins:
To use a mixin, simply inherit from it alongside other view classes when defining your view. For instance, if you have a view that requires authentication and uses Django’s generic `DetailView`, you can do the following:
```python from django.views.generic import DetailView from .models import YourModel from .mixins import AuthMixin class AuthenticatedDetailView(AuthMixin, DetailView): model = YourModel template_name = 'your_template.html' ```
In this example, the `AuthMixin` is mixed into the `AuthenticatedDetailView`, allowing you to reuse the authentication check logic across multiple views.
Benefits of Mixins:
Django mixins offer several advantages, including:
- Code Reusability: You can encapsulate common functionality in mixins and reuse them in multiple views, reducing code duplication.
- Modularity: Mixins promote modular code, making it easier to maintain, test, and extend your views.
- Flexibility: You can mix and match different mixins to compose views with varying functionalities, adapting to your project’s specific needs.
- Cleaner Code: By separating concerns into mixins, your views become more focused and easier to understand.
Django mixins are a valuable tool for building flexible and modular views in Django. They promote code reusability, modularity, and cleaner code, making your development process more efficient and your codebase more maintainable.
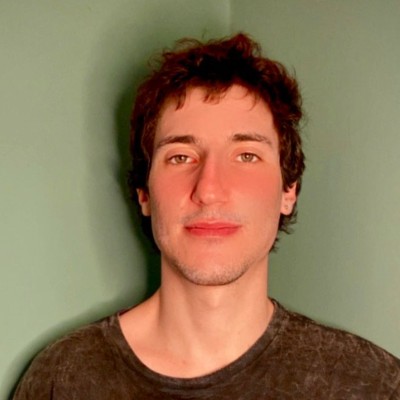
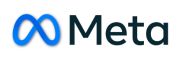