The Ultimate Guide: Crafting Mobile Apps with Django’s Magic
Django is a high-level Python web framework that promotes rapid development and clean, pragmatic design. While it’s mainly known for creating web applications, you can also use Django to serve data to mobile apps. With the rise of smartphones and the app-centric way of consuming content, understanding how to pair Django with mobile app development can be an invaluable skill.
Table of Contents
In this blog post, we’ll explore how you can use Django to build mobile apps, focusing on two main approaches: creating RESTful APIs for mobile clients and using web-view based mobile apps.
1. Using Django to Create RESTful APIs for Mobile Apps
A RESTful API (Representational State Transfer) provides a way for mobile apps to fetch and send data to a server. Mobile apps built on native platforms (like Android or iOS) can interact with Django by fetching or sending data through the API endpoints.
1.1 Building a simple Todo API
- Setting up the Django Project and App:
```bash django-admin startproject mobileapi cd mobileapi python manage.py startapp todos ```
- Design the Todo Model:
In `todos/models.py`:
```python from django.db import models class Todo(models.Model): title = models.CharField(max_length=200) completed = models.BooleanField(default=False) def __str__(self): return self.title ```
- Create a Serializer:
Serializers allow complex data types, such as Django models, to be converted to easily renderable types like JSON.
Install the Django REST framework first:
```bash pip install djangorestframework ```
In `todos/serializers.py`:
```python from rest_framework import serializers from .models import Todo class TodoSerializer(serializers.ModelSerializer): class Meta: model = Todo fields = ['id', 'title', 'completed'] ```
- Design the API views:
In `todos/views.py`:
```python from rest_framework import generics from .models import Todo from .serializers import TodoSerializer class TodoList(generics.ListCreateAPIView): queryset = Todo.objects.all() serializer_class = TodoSerializer class TodoDetail(generics.RetrieveUpdateDestroyAPIView): queryset = Todo.objects.all() serializer_class = TodoSerializer ```
- Wire up the URLs:
In `todos/urls.py`:
```python from django.urls import path from . import views urlpatterns = [ path('todos/', views.TodoList.as_view(), name='todo-list'), path('todos/<int:pk>/', views.TodoDetail.as_view(), name='todo-detail'), ] ```
Mobile apps can now make HTTP requests to these endpoints, fetch the list of todos, or interact with individual todo items.
2. Web-View Based Mobile Apps
Another approach is to use Django to serve mobile-optimized web pages that can be embedded in mobile apps using “web views.” Frameworks like React Native or Flutter have mechanisms to embed web content.
2.1 Building a mobile-optimized web app with Django and WebView in React Native
- Design a mobile-optimized template in Django:
Using Bootstrap or another responsive design library can help. Let’s create a simple mobile-optimized todo list.
- Set up React Native with WebView:
Initialize a new React Native app and add the WebView component.
```bash npx react-native init MobileWebApp cd MobileWebApp npm install react-native-webview ```
- Embed the Django web page in React Native WebView:
```jsx import { WebView } from 'react-native-webview'; function App() { return ( <WebView source={{ uri: 'http://your-django-server-url/todos/' }} /> ); } ```
This approach lets you use Django’s ORM and templating engine, but you’ll be somewhat limited in terms of leveraging native mobile features.
Conclusion
Django’s versatility makes it a powerful back-end tool not just for web apps but also for mobile. Whether you’re building native mobile apps that require a robust backend and API or web-view based mobile apps that leverage Django’s templating engine, Django has got you covered. While there’s no one-size-fits-all, understanding the strengths and limitations of each approach will allow you to make an informed decision based on your project’s needs.
Table of Contents
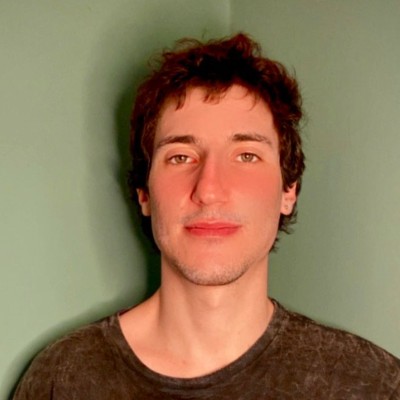
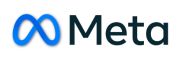