Mastering Django Multi-Tenancy: The Ultimate Guide for Multi-Client Success
In the dynamic world of web development, the concept of multi-tenancy has become increasingly relevant, especially for businesses aiming to provide services to a diverse client base through a single application instance. Django, a high-level Python web framework, emerges as a robust solution for developing such multi-tenant applications. This blog post delves into the essence of multi-tenancy in Django, offering insights and practical examples to guide developers in building efficient, scalable multi-tenant applications. You can hire Django Developers for your projects to ensure greater success.
Table of Contents
1. What is Multi-tenancy?
Multi-tenancy refers to a software architecture where a single instance of the software runs on a server, serving multiple tenants. A tenant is a group of users who share a common access with specific privileges to the software instance. In simpler terms, think of it as an apartment building where each tenant lives in a separate unit but shares the building infrastructure.
Example:
Imagine an online project management tool used by various companies. Each company, although using the same application, has its own data, users, and customization – these companies are the tenants.
2. Why Django for Multi-tenancy?
Django, with its modular architecture, extensive libraries, and robust ORM (Object-Relational Mapping), is an ideal choice for developing multi-tenant applications. It simplifies handling different data schemas and tenant-specific data management, crucial in a multi-tenant architecture.
Key Features:
– Scalability: Django’s scalability is apt for handling the growing number of tenants.
– Security: Django provides built-in security features that are essential for protecting tenant data.
– Flexibility: Django’s flexibility allows customization for tenant-specific needs.
3. Building a Multi-tenant Application in Django
Step 1: Tenant Isolation
The first step is to decide the level of isolation between tenants. There are primarily three approaches:
- Shared Database, Shared Schema: All tenants share the same database and schema. Data is differentiated by a tenant ID.
- Shared Database, Separate Schemas: Tenants share the same database but have separate schemas.
- Separate Databases: Each tenant has its own database.
For simplicity and efficiency, we will focus on the “Shared Database, Shared Schema” approach.
Step 2: Setting Up Django Environment
Ensure you have Django installed. If not, you can install it using pip:
```bash pip install django ```
Step 3: Tenant Identification
Use Django’s middleware to identify tenants. You can identify tenants based on the domain, subdomain, or a URL path. Here’s an example of a middleware that identifies tenants by subdomain:
```python # middleware.py from django.http import Http404 def get_tenant(request): host = request.get_host().split('.') if len(host) < 3: raise Http404("Tenant not found") return host[0] class TenantMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): request.tenant = get_tenant(request) return self.get_response(request) ```
Step 4: Database Models
Design your database models to include a tenant identifier. For example:
```python from django.db import models class Project(models.Model): tenant_id = models.CharField(max_length=100) name = models.CharField(max_length=100) # other fields ```
Step 5: Querying Tenant Data
When querying data, ensure you filter based on the tenant ID:
```python projects = Project.objects.filter(tenant_id=request.tenant) ```
Step 6: Deploying and Testing
Once your application is ready, deploy it and conduct thorough testing to ensure each tenant’s data is isolated and the application functions as intended.
4. Best Practices and Considerations
– Data Security: Implement rigorous security measures to protect tenant data.
– Performance Optimization: Optimize queries and use caching to enhance performance.
– Scalable Architecture: Design your application to be scalable as the number of tenants grows.
Conclusion
Building a multi-tenant application with Django is a smart choice for developers looking to create scalable, efficient, and secure web applications for multiple clients. By following the steps and best practices outlined in this guide, you can embark on your journey to developing a robust multi-tenant application.
For more insights and advanced techniques in Django multi-tenancy, consider exploring these resources:
- Django’s official documentation
- Advanced multi-tenancy in Django
- Scaling multi-tenant applications
- Django packages for multi-tenancy
You can check out our other blog posts to learn more about Django. We bring you a complete guide titled Discover How Django Is Revolutionizing the Blogging World along with the Master Real-Time Communication in Django Apps with WebSockets and Mastering Django Admin: Your Guide to Efficient Web Application Management which will help you understand and gain more insight into the Django programming language.
Table of Contents
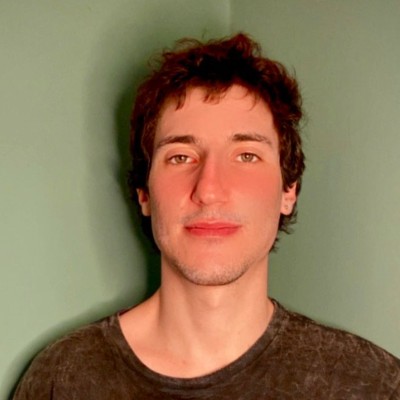
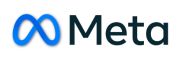