How integrate Django with a NoSQL database like MongoDB?
Integrating Django with a NoSQL database like MongoDB can be a useful choice when you have data that doesn’t fit well into a traditional relational database schema. To achieve this integration, you can use Django’s `django-nonrel` project along with the `django-mongodb-engine` driver. Here’s a step-by-step guide on how to do it:
- Install Required Packages:
Start by installing the necessary packages:
```bash pip install django-nonrel pip install django-mongodb-engine ```
- Configure Your Django Project:
In your Django project’s settings, make the following changes:
```python DATABASES = { 'default': { 'ENGINE': 'django_mongodb_engine', 'NAME': 'your_database_name', } } INSTALLED_APPS = ( # ... 'djangotoolbox', 'mongodb_engine', # ... ) ```
Replace `’your_database_name’` with the name of your MongoDB database.
- Define Models:
You can create Django models as you would for a relational database, but keep in mind that NoSQL databases like MongoDB are schema-less, allowing for flexible data structures. Here’s an example:
```python from django.db import models class Product(models.Model): name = models.CharField(max_length=100) description = models.TextField() price = models.DecimalField(max_digits=10, decimal_places=2) ```
- Migrate Your Models:
Run the following command to create collections for your models in MongoDB:
```bash python manage.py syncdb ```
- Query and Interact with MongoDB:
You can use Django’s query API to interact with MongoDB just like you would with a traditional database. However, keep in mind that some advanced MongoDB features, like complex aggregations, may require using MongoDB’s native query language.
```python # Query all products products = Product.objects.all() # Filter products expensive_products = Product.objects.filter(price__gte=100) # Create a new product new_product = Product(name="New Product", description="A new product", price=50) new_product.save() ```
By following these steps, you can integrate Django with MongoDB, allowing you to leverage the flexibility and scalability of a NoSQL database while still benefiting from Django’s robust development framework. This can be particularly useful when dealing with data that doesn’t fit neatly into a relational model or when you need to handle large volumes of unstructured or semi-structured data.
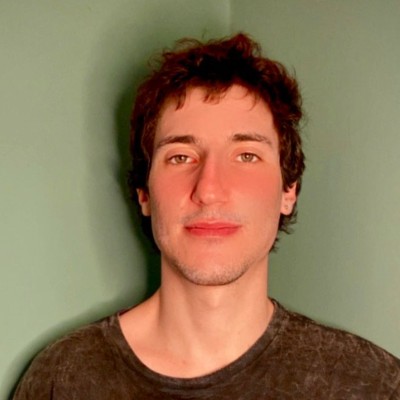
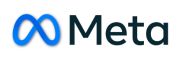