How to implement OAuth2 authentication in Django?
To implement OAuth2 authentication in a Django REST framework (DRF) API, you can leverage a package called `django-oauth-toolkit`. OAuth2 is a widely used protocol for securing APIs by allowing third-party applications to access user data without exposing user credentials. Here’s how you can set it up:
- Install `django-oauth-toolkit`:
You can install it using pip:
``` pip install django-oauth-toolkit ```
- Add `oauth2_provider` to your Django apps:
In your project’s `settings.py` file, add `’oauth2_provider’` to the `INSTALLED_APPS` list.
- Migrate the Database:
Run the following command to create the necessary database tables for `oauth2_provider`:
``` python manage.py migrate ```
- Configure OAuth2 Settings:
In your `settings.py`, configure the OAuth2 settings. For example:
```python OAUTH2_PROVIDER = { 'SCOPES': { 'read': 'Read scope', 'write': 'Write scope', } } ```
- Create OAuth2 Applications:
OAuth2 requires applications to be registered. You can create them using Django admin or programmatically. Each application will have a client ID and a client secret.
- Apply Authentication Classes:
In your DRF views or viewsets, you can apply OAuth2 authentication classes like this:
```python from oauth2_provider.contrib.rest_framework import OAuth2Authentication class YourApiView(APIView): authentication_classes = [OAuth2Authentication] permission_classes = [IsAuthenticated] ```
- Token Retrieval:
Clients need to obtain access tokens by following the OAuth2 flow. This typically involves sending a POST request with client credentials and user authorization.
- Use Access Tokens:
In your API views, you can now check for valid access tokens to secure your endpoints. The `IsAuthenticated` permission will handle this for you.
- Refresh Tokens (Optional):
You can configure OAuth2 to issue refresh tokens, allowing clients to obtain new access tokens without user intervention.
OAuth2 is a powerful and flexible authentication mechanism for securing your Django REST API. By following these steps, you can implement OAuth2 authentication and provide secure access to your resources for third-party applications while keeping user credentials safe.
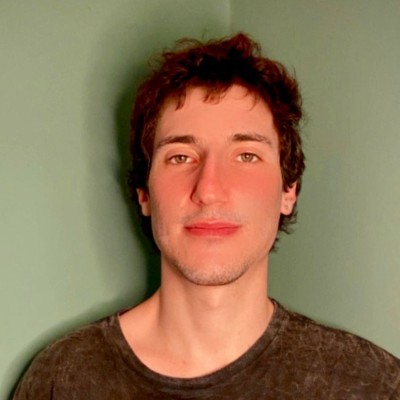
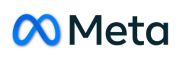