How to optimize database queries in Django?
Optimizing database queries in Django is crucial for ensuring the efficiency and performance of your web application, especially as your database grows in size and complexity. Here are several techniques to optimize database queries in Django:
- Use Django’s Querysets:
– Django provides Querysets, which allow you to construct complex database queries using Pythonic syntax. Leverage the power of filter(), exclude(), and annotate() methods to retrieve only the data you need.
- Indexing:
– Identify fields that are frequently used in WHERE clauses, and apply database indexes to them. This significantly speeds up query performance by allowing the database to quickly locate matching rows.
- Limit Fields in SELECT:
– When querying, select only the fields that you actually need. Avoid using select_related() or prefetch_related() if they’re not necessary, as these can lead to unnecessary data retrieval.
- Caching:
– Implement caching mechanisms, such as Django’s built-in caching framework or third-party solutions like Redis, to store frequently used query results in memory. This reduces the need to query the database repeatedly.
- Database Optimization Tools:
– Utilize database-specific tools like Django Debug Toolbar for profiling and analyzing query performance. These tools help identify slow queries and potential optimizations.
- Use Database Profiling:
– Profiling tools like Django Silk can help you identify bottlenecks and slow queries in your application. Use this information to fine-tune your database queries.
- Database Sharding:
– If your application experiences high traffic and data growth, consider database sharding. It involves distributing data across multiple databases or servers to improve scalability.
- Denormalization:
– In some cases, denormalizing your database schema by storing redundant data can reduce the need for complex joins and speed up queries. However, use this technique judiciously, as it may introduce data integrity challenges.
- Database Maintenance:
– Regularly perform database maintenance tasks, such as vacuuming or optimizing database tables, to keep your database in good shape.
- Use Connection Pooling:
– Employ connection pooling to reuse and manage database connections efficiently. Tools like PgBouncer or Gunicorn can help with this.
By applying these best practices and monitoring your application’s database queries, you can optimize performance, reduce latency, and ensure a responsive user experience, even as your Django project grows in complexity and scale.
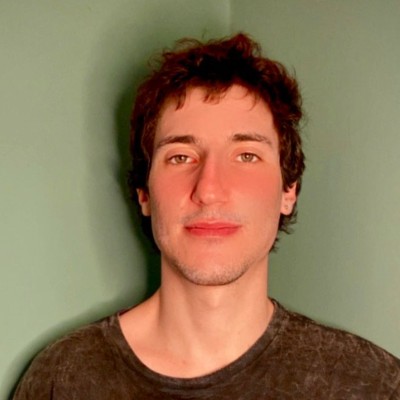
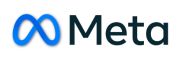