How to perform CRUD (Create, Read, Update, Delete) operations in Django?
Performing CRUD (Create, Read, Update, Delete) operations in Django is a fundamental aspect of building web applications that interact with databases. Django provides an elegant and efficient way to handle these operations through its Object-Relational Mapping (ORM) and views. Here’s an overview of how to perform CRUD operations in Django:
- Create (C):
– To create new database records, define a model class in Django, which represents the structure of the data you want to store.
– Define a form to capture user input, typically using Django’s `ModelForm` or custom form classes.
– In your view function, validate the form data, create a new instance of the model with the validated data, and save it to the database using the `save()` method.
– Return an appropriate response, such as a confirmation message or a redirection to a detail view.
- Read (R):
– Reading data from the database in Django is straightforward. Define a view that queries the database using the model’s manager (e.g., `MyModel.objects.all()` or `MyModel.objects.get(pk=1)`).
– Pass the retrieved data to a template for rendering.
– In the template, use template tags to display the data to the user.
- Update (U):
– To update existing records, create a form similar to the one used for the create operation, pre-filled with the data to be edited.
– In the view function, validate the form data and update the corresponding database record using the `save()` method.
– Redirect the user to a detail view or display a success message.
- Delete (D):
– To delete records, define a view that retrieves the object to be deleted and calls the `delete()` method on it.
– Consider adding confirmation dialogs or implementing permission checks to prevent accidental deletions.
– Redirect the user to a list view or display a success message.
Django’s ORM and class-based views make it relatively simple to implement these CRUD operations. Additionally, Django provides built-in security measures to protect against common vulnerabilities such as SQL injection and cross-site request forgery (CSRF). Properly handling CRUD operations is crucial for creating robust and user-friendly web applications with Django.
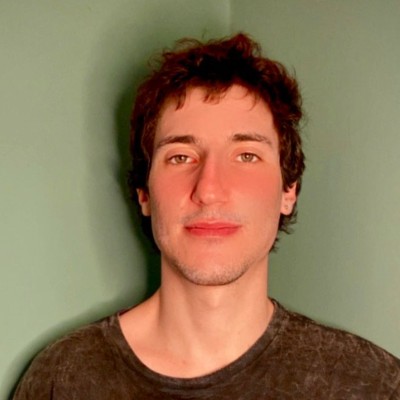
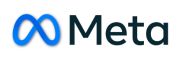