The Need for Speed: Enhancing Performance with Django
Django is a highly powerful and flexible Python web framework that enables developers to create robust web applications. But like all tools, it requires skillful handling to truly shine, especially in terms of performance. This skillful handling can often be best achieved when you hire Django developers who understand the intricacies of the framework.
In this blog post, we’ll delve into the world of Django performance optimization. We’ll present key strategies to boost your web app’s speed and demonstrate these strategies with relevant examples. These examples provide practical insight into the optimizations you can expect when you hire Django developers experienced in performance enhancement. By integrating these strategies into your development process, you can significantly improve your application’s speed and response times.
Understanding Django Performance
Performance optimization should not be an afterthought. In Django, it involves various aspects, such as database optimization, effective caching, application-level optimization, middleware ordering, and more. By integrating these strategies into your development process, you can significantly improve your application’s speed and response times.
Database Optimization
The way Django interacts with the database can significantly influence your web application’s performance. Here are some tips to optimize your database interactions:
Efficient Querying
The Django ORM makes database interactions incredibly simple. However, carelessness can lead to inefficient queries, causing slow page loads and a sluggish app.
Here’s an example: if you are retrieving a list of books and their authors from a database, you might have a loop that gets each book’s author:
```python books = Book.objects.all() for book in books: print(book.author.name) ```
The problem is that each iteration triggers a separate database query to fetch the author, leading to what we call the “N+1” queries problem.
To resolve this, Django provides `select_related` and `prefetch_related` methods.
`select_related` is used when you have a `ForeignKey` or `OneToOneField` in your models. Here’s how to use it:
```python books = Book.objects.select_related('author').all() for book in books: print(book.author.name) ```
Here, only one SQL query is executed to fetch all the data, which boosts the performance significantly.
`prefetch_related`, on the other hand, is ideal for `ManyToManyField` and reverse `ForeignKey` fields:
```python authors = Author.objects.prefetch_related('books').all() for author in authors: print([book.title for book in author.books.all()]) ```
Database Indexing
Indexing fields that are frequently queried can significantly speed up read operations. Django allows adding database indexes to your models using `db_index=True` or `indexes` options in your model’s Meta class.
```python class Book(models.Model): title = models.CharField(max_length=200, db_index=True) ... ```
Or using Meta class,
```python class Book(models.Model): title = models.CharField(max_length=200) class Meta: indexes = [ models.Index(fields=['title']), ] ```
Middleware Optimization
Middleware classes run before view functions, and the order in which they are listed in `MIDDLEWARE` setting can impact your app’s performance. Put middleware classes that are hit more often at the top of the list to avoid running unnecessary middleware.
Caching
Caching is a critical strategy to improve your Django application’s performance. By storing the results of expensive or frequent operations, you can serve subsequent requests faster.
Django provides a robust caching framework that you can use at various levels: per-view, template fragment caching, or low-level caching.
Per-View Caching
Django provides a decorator called `cache_page` that you can use to cache the entire output of a view:
```python from django.views.decorators.cache import cache_page @cache_page(60 * 15) # cache for 15 minutes def my_view(request): ... ```
Template Fragment Caching
If you have a part of your template that takes time to render but doesn’t change often, you can use Django’s template fragment caching:
```html {% load cache %} {% cache 500 sidebar %} <div> <!-- expensive content here --> </div> {% endcache %} ```
Low-Level Cache API
For more control, you can use Django’s low-level cache API to store values:
```python from django.core.cache import cache def expensive_calculation(): result = cache.get('my_key') if result is None: result = do_expensive_calculation() cache.set('my_key', result, 60 * 5) # cache for 5 minutes return result ```
Application-Level Optimization
Use `values` and `values_list` When Possible
When you only need a subset of data from the database, you can use `values` or `values_list` to avoid loading whole model instances:
```python titles = Book.objects.values_list('title', flat=True) ```
Avoiding Python `len()` on QuerySets
When determining the number of records in a queryset, use Django’s `count()` method, which translates to a SQL `COUNT`, rather than Python’s `len()` function which loads objects into memory.
```python book_count = Book.objects.count() # Efficient book_count = len(Book.objects.all()) # Inefficient ```
Conclusion
Performance optimization in Django is a broad field with many avenues. To truly make your application fast, thoughtful considerations should be made during the entire development process, not just as a final step. This is where hiring Django developers with a strong understanding of performance optimization can make a significant difference.
In this blog post, we’ve explored database optimization, middleware ordering, caching, and application-level optimization. But, there’s more to Django performance optimization, including advanced techniques such as asynchronous views, using a Content Delivery Network (CDN), static files compression, and so on. These advanced techniques are often well-handled by seasoned Django developers.
Remember, performance optimization is a continuous process, and each optimization must be measured to ensure it has the desired impact. This is another area where Django developers shine, using Django’s debugging tools and logging to identify bottlenecks in your application effectively.
With the techniques explored in this blog post and a team of skilled Django developers, you’re well on your way to creating faster, more efficient Django applications. Happy coding!
Table of Contents
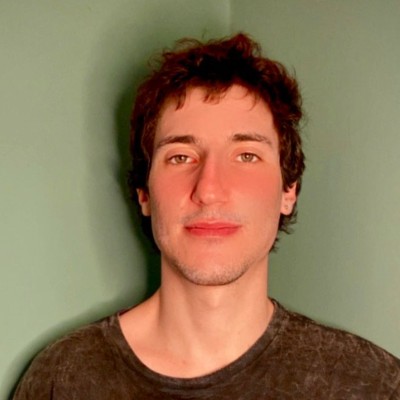
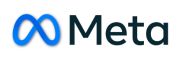