Django and Progressive Web Apps: Creating Offline-Ready Web Applications
In today’s fast-paced digital world, web applications need to be more than just responsive and user-friendly. They should also be capable of providing a seamless user experience, even when offline. Progressive Web Apps (PWAs) are the answer to this challenge, and when combined with Django, a powerful Python web framework, they can take your web application to the next level.
Table of Contents
In this comprehensive guide, we will walk you through the process of creating a Django-based Progressive Web App, complete with code samples, practical examples, and step-by-step instructions. By the end of this tutorial, you will have the knowledge and tools to transform your Django web application into an offline-ready, highly engaging PWA.
1. What is a Progressive Web App (PWA)?
Progressive Web Apps are web applications that leverage modern web technologies to provide an app-like experience to users, whether they are using a desktop browser or a mobile device. PWAs offer several key advantages:
- Offline Access: PWAs can work offline or with a poor internet connection, ensuring that users can access your application’s content and features at all times.
- Responsive Design: They adapt to various screen sizes and devices, providing a consistent experience across platforms.
- Fast Loading: PWAs are known for their quick loading times, enhancing user engagement and retention.
- Installable: Users can add PWAs to their home screens, just like native apps, eliminating the need for app store downloads.
- Push Notifications: You can send push notifications to users, increasing user engagement and retention.
Now, let’s dive into the steps required to create a Django-powered Progressive Web App.
2. Setting Up Your Django Project
Before we start adding PWA features to our Django project, make sure you have a Django project up and running. If you don’t have one, you can create a new project by running the following commands:
bash pip install django django-admin startproject mypwa cd mypwa
Next, create a Django app within your project:
bash python manage.py startapp myapp
Ensure you have the necessary database tables set up and create some sample data to work with.
3. Adding PWA Functionality to Django
To turn your Django project into a Progressive Web App, you’ll need to install a few packages and make some configurations. Here are the steps to follow:
3.1. Install the django-pwa Package
The django-pwa package simplifies the process of adding PWA functionality to your Django project. You can install it using pip:
bash pip install django-pwa
3.2. Configure django-pwa in Your Django Project
In your project’s settings (usually located in settings.py), add ‘pwa’ to your INSTALLED_APPS:
python INSTALLED_APPS = [ # ... 'pwa', ]
Next, define the configuration settings for django-pwa. Add the following to your settings:
python PWA_APP_NAME = "My PWA App" PWA_APP_DESCRIPTION = "A fantastic Progressive Web App" PWA_APP_THEME_COLOR = "#007bff" PWA_APP_BACKGROUND_COLOR = "#ffffff" PWA_APP_DISPLAY = 'standalone' PWA_APP_SCOPE = '/' PWA_APP_ORIENTATION = 'portrait' PWA_APP_START_URL = '/' PWA_APP_ICONS = [ { 'src': '/static/images/icon.png', 'sizes': '160x160' } ] PWA_APP_ICONS_APPLE = [ { 'src': '/static/images/apple-icon.png', 'sizes': '160x160' } ] PWA_SERVICE_WORKER_PATH = os.path.join(BASE_DIR, 'static/js', 'serviceworker.js')
These settings define various aspects of your PWA, including its name, description, theme color, and more.
3.3. Create a Service Worker
A Service Worker is a JavaScript file that runs in the background, enabling your PWA to work offline and provide other advanced features. Create a new file named serviceworker.js in your project’s static/js directory:
javascript // serviceworker.js self.addEventListener('fetch', (event) => { event.respondWith( caches.match(event.request) .then((response) => { return response || fetch(event.request); }) ); });
3.4. Configure URL Patterns
In your Django project, you’ll need to configure URL patterns to specify which URLs should be cached by the Service Worker. Open your urls.py file and add the following:
python from django.urls import path from django.views.decorators.cache import cache_page from django.conf import settings from django.conf.urls.static import static from pwa import views as pwa_views urlpatterns = [ # ... path('offline/', cache_page(settings.PWA_APP_NAME)(pwa_views.OfflineView.as_view())), ] if settings.DEBUG: urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
In this example, we’ve added a URL pattern for an offline page. You can customize this URL and view to fit your project’s needs.
3.5. Include PWA Elements in Your HTML Templates
To make your Django templates PWA-compatible, you need to include some essential elements. In your base HTML template, add the following:
html {% load pwa %} <!DOCTYPE html> <html lang="en"> <head> <!-- ... --> {% progressive_web_app_meta %} </head> <body> <!-- ... --> {% progressive_web_app_script %} </body> </html>
These template tags generate the necessary meta tags and scripts to enable PWA functionality in your application.
4. Testing Your Django PWA
With the PWA functionality integrated into your Django project, it’s time to test your application as a Progressive Web App. Follow these steps:
4.1. Run the Development Server
Start your Django development server:
bash python manage.py runserver
4.2. Access Your PWA
Open a web browser and navigate to your Django application. You can use the development server’s default address, typically http://localhost:8000.
4.3. Enable PWA Features
To test PWA features, use the web browser’s developer tools. In Google Chrome, for instance, open the DevTools, go to the “Application” tab, and check the “Service Workers” and “Manifest” sections. You should see your PWA listed.
4.4. Test Offline Functionality
To test offline functionality, you can use the “Offline” checkbox in the DevTools under the “Service Workers” section. Enable this option and refresh your PWA. You should still be able to access the cached content even when offline.
5. Deploying Your Django PWA
Once you’ve thoroughly tested your Django PWA, it’s time to deploy it to a production server. Here are the general steps to follow:
5.1. Choose a Hosting Provider
Select a hosting provider that suits your project’s needs. Popular options for Django applications include Heroku, AWS, DigitalOcean, and PythonAnywhere.
5.2. Configure Your Production Environment
Set up a production environment by following your hosting provider’s guidelines. This typically involves configuring a web server (e.g., Nginx or Apache), setting up a database, and ensuring security measures.
5.3. Deploy Your PWA
Use the hosting provider’s tools or command-line interfaces to deploy your Django PWA. You’ll need to upload your code, configure database settings, and set environment variables.
5.4. Set Up HTTPS
For a PWA to work correctly, it must be served over HTTPS. Obtain an SSL certificate and configure your web server to use HTTPS.
5.5. Monitor and Maintain Your PWA
Regularly monitor your PWA in the production environment. Set up error tracking, performance monitoring, and backups to ensure the smooth operation of your application.
Conclusion
Progressive Web Apps offer a compelling way to enhance the user experience of your Django web application. By following the steps outlined in this guide, you can create an offline-ready PWA that provides fast loading times, responsive design, and the ability to engage users even when they are offline.
Take your Django project to the next level by harnessing the power of PWAs and giving your users a seamless and enjoyable experience, no matter where they are or what device they are using. Start today and transform your web application into a Progressive Web App that stands out in the digital landscape. Happy coding!
Table of Contents
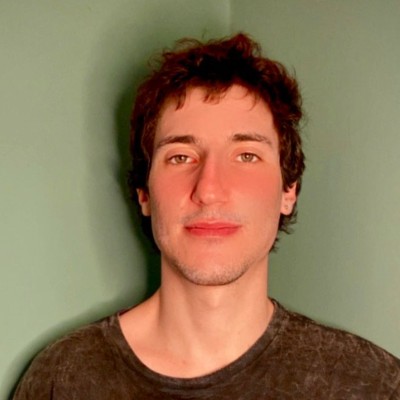
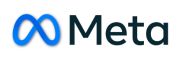