What is the REST framework, and how to use it to build APIs in Django?
Django REST framework (DRF) is a powerful and versatile toolkit for building Web APIs in Django applications. It extends Django’s capabilities to make it easy to create APIs that can handle various HTTP methods, serialize data, and authenticate users. Here’s an explanation of what Django REST framework is and how to use it to build APIs:
What is the Django REST framework?
Django REST framework is a third-party library that simplifies the process of building web APIs in Django. It provides a set of tools and conventions for creating, testing, and documenting APIs, making it an excellent choice for developing RESTful services. DRF includes features like serialization (converting complex data types like Django models to Python data types), authentication, view classes, and URL routing specifically tailored for APIs.
Using Django REST framework to Build APIs:
- Installation: Begin by installing Django REST framework using pip:
``` pip install djangorestframework ```
- Configuration: Add `’rest_framework’` to the `INSTALLED_APPS` list in your project’s `settings.py` file to enable DRF.
- Serializers: In DRF, serializers are used to convert complex data types (e.g., Django models) into JSON or XML data that can be easily rendered into content types suitable for client consumption. Create serializer classes by extending `serializers.ModelSerializer` for model-based serializers or `serializers.Serializer` for custom data structures.
- Views and ViewSets: DRF provides a range of view classes that handle various HTTP methods (GET, POST, PUT, DELETE). You can use `APIView` for custom views or `ModelViewSet` for views that correspond to Django models. These views are similar to Django’s class-based views but tailored for API endpoints.
- URL Routing: Define URL patterns for your API using DRF’s router and viewset classes. This allows you to automatically generate URLs for your API endpoints.
- Authentication and Permissions: DRF includes built-in support for various authentication methods like Token Authentication, Session Authentication, and more. You can also define custom permissions to control access to your API views.
- Testing: DRF provides a powerful testing framework that allows you to write test cases for your APIs. This ensures that your API endpoints behave as expected and helps maintain code quality.
- Documentation: DRF includes automatic generation of interactive and browsable API documentation using the `BrowsableAPIRenderer`. This makes it easier for developers to explore and understand your API.
By following these steps and leveraging the features provided by Django REST framework, you can quickly and efficiently build robust and well-documented APIs for your Django applications. DRF’s flexibility, extensive documentation, and active community make it a popular choice for building RESTful APIs in Django projects.
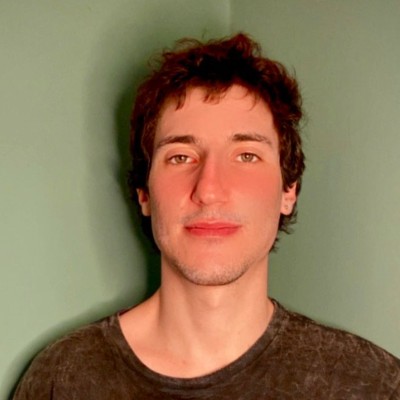
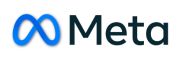