Django Web Development: Building Scalable and Secure Applications
When it comes to web development, choosing the right framework can make or break your project. Among the plethora of frameworks available in the market, Django has emerged as a popular choice for developers worldwide. This Python-based framework is lauded for its simplicity, flexibility, and robustness. If you’re considering building a scalable and secure web application, it’s time to hire Django developers who can help you leverage these qualities.
Why Django?
Before we dive into how to build scalable and secure applications, let’s take a moment to understand why Django is a solid choice for web development.
Django follows the “batteries-included” philosophy, meaning it comes with a wide array of functionalities out of the box. The framework offers various tools for database schema migration, templating, URL routing, and more. Plus, it has excellent support for a wide variety of databases and a robust user authentication system. All these aspects make Django an excellent option to create complex, data-driven websites.
Moreover, Django is built on Python – a language known for its simplicity and readability. This makes the framework easy to learn and use, especially for newcomers in the field. Consequently, when you hire Django developers, you can expect them to ramp up quickly and contribute to your project effectively.
Building Scalable Django Applications
Scalability refers to an application’s ability to handle an increase in workload or expand in response to increased demand. When building a web application, it’s crucial to consider scalability from the outset.
In Django, scalability can be addressed through various strategies. One approach is horizontal scaling, where you add more servers to your system to manage the increased load. Django, with its stateless nature, supports this form of scaling very effectively. Every request can be processed independently, allowing for the addition of new servers without significant changes to the existing architecture.
Another approach to improve scalability when you hire Django developers is to implement caching. Django comes with a robust caching framework that allows you to store dynamic web pages, thereby reducing the server load and increasing site performance. It supports various cache backends, such as Memcached and Database caching, giving you the flexibility to choose based on your requirements.
Lastly, effective database optimization is also crucial for scalability. Django offers several tools for database optimization, such as database indexing, efficient querying, and denormalization. It also provides an ORM (Object-Relational Mapping) that allows developers to interact with the database, like they would with SQL. In fact, Django’s ORM is one of the reasons to hire Django developers, as it makes handling databases more manageable and less prone to errors.
How to Build Scalable and Secure Django Applications
Building scalable and secure applications requires a strategic approach that includes proper coding practices, architecture design, and utilization of Django’s robust features. Let’s dive deeper.
Building Scalable Django Applications
- Database Optimization
Django’s ORM allows you to handle database operations using Python code. But inefficient querying can significantly slow down your application. Let’s take an example:
```python # Inefficient querying authors = Author.objects.all() for author in authors: print(author.book_set.count()) # Efficient querying authors = Author.objects.annotate(num_books=Count('book')) for author in authors: print(author.num_books)
The first code block hits the database each time it counts books for an author, which can be slow with a large dataset. The second block uses the Django ORM’s `annotate` function to perform the same operation in a single database query, thereby improving scalability.
- Caching
To further improve scalability, consider caching. Django’s caching framework lets you cache entire rendered HTML pages or parts of them. Consider this example where we cache a view that takes long to render:
```python from django.views.decorators.cache import cache_page @cache_page(60 * 15) # cache for 15 minutes def my_view(request):
- Using a Content Delivery Network (CDN)
When your user base is distributed worldwide, using a CDN can improve the application’s response times. Django’s `django-storages` library allows integrating your app with CDNs like Amazon S3, Google Cloud Storage, and others.
Building Secure Django Applications
- Protection Against Cross-Site Scripting (XSS)
Django escapes variables by default in its template system to protect against XSS attacks. Here’s an example:
```python {{ variable }}
The above code will auto-escape any HTML code contained in `variable`.
- Cross-Site Request Forgery (CSRF) Protection
Django has built-in middleware for CSRF protection. To use it in your templates, you simply include a CSRF token inside your forms like so:
```html <form method="post">{% csrf_token %}
- SQL Injection Protection
Django’s QuerySet API protects against SQL injection by default because it escapes all variables that go into the database automatically. Consider this example:
```python Entry.objects.get(pub_date__year=2005)
Even if the year variable is provided by a user and is a string that contains SQL, Django will properly quote it to avoid a SQL Injection attack.
- Secure Password Handling
Django’s authentication framework provides a secure way to handle user passwords. It stores password hashes instead of the actual passwords, ensuring data security even in the event of a data breach.
- Using HTTPS
You can configure Django to use HTTPS for all interactions, ensuring that all data sent between the user and your server is encrypted. To enforce HTTPS, you can use Django’s `SecurityMiddleware`:
```python SECURE_SSL_REDIRECT = True
With this configuration, if a user accesses your site over HTTP, they’ll be redirected to HTTPS.
Ensuring Security in Django Applications
Securing your web applications is paramount in today’s digital landscape. Fortunately, Django shines in this area as well, offering multiple built-in tools and mechanisms to help create secure websites.
One notable feature of Django is its inbuilt protection against common security vulnerabilities, like Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and SQL Injection. When you hire Django developers, they don’t have to spend extra time securing your application against these attacks, as the framework automatically handles these issues.
Django also offers a robust user authentication system, providing mechanisms to handle user accounts, cookies, sessions, and other elements associated with user authentication. Furthermore, Django enables HTTPS for the entire site, providing an additional layer of security.
Lastly, Django’s ‘middleware’ system allows developers to process requests and responses globally before they reach the view and after they leave it. This can be effectively used to apply security measures across the entire application, such as implementing authentication, authorization, session management, and more.
Conclusion
Django’s ability to build scalable and secure web applications is largely due to its robust set of features and mechanisms. However, to effectively leverage these capabilities, it’s essential to hire Django developers who are proficient in the framework. They can understand your business requirements, identify potential scalability and security issues, and utilize Django’s tools effectively to create a successful web application.
As the demand for efficient, scalable, and secure web applications continues to grow, Django web development is becoming increasingly significant. So, if you’re considering building a web application, hire Django developers and let Django’s power and simplicity turn your idea into a robust, scalable, and secure reality.
Table of Contents
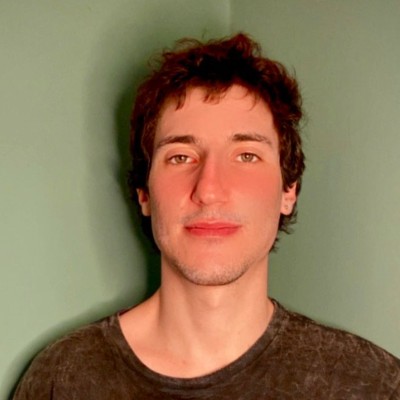
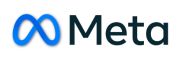