Django Q & A
How to schedule tasks in Django using Celery?
Scheduling tasks in Django using Celery is a common practice to offload time-consuming or recurring tasks from the main application thread, ensuring efficient performance and responsiveness. Celery is a distributed task queue framework that allows you to execute tasks asynchronously and on a predefined schedule. Here’s a step-by-step guide on how to schedule tasks in Django using Celery:
- Install Celery: Start by installing Celery and a message broker such as RabbitMQ or Redis. You can install Celery using pip:
``` pip install celery ```
- Configure Celery: In your Django project, create a Celery configuration file, typically named `celery.py` or `celery_config.py`. Configure Celery to use your chosen message broker and specify the result backend (usually a database or Redis).
- Create Celery Tasks: Define the tasks you want to schedule as Python functions in your Django project. Decorate these functions with `@celery.task` to indicate that they are Celery tasks.
- Schedule Tasks: To schedule tasks, you can use Celery’s built-in task scheduling, which relies on Celery Beat, a scheduler included with Celery. Configure Celery Beat by specifying the schedule for each task in your Celery configuration.
- Start Celery Workers and Beat: Start Celery workers to process tasks and Celery Beat to manage the schedule. You can do this using the following command:
``` celery -A your_project_name worker --loglevel=info celery -A your_project_name beat --loglevel=info ```
- Monitor and Debug: Monitor task execution and debug any issues by checking Celery logs and the Django admin interface, which provides visibility into task status and results.
- Error Handling: Implement error handling and retry mechanisms for tasks that may fail, ensuring robust task execution.
By following these steps, you can leverage Celery to schedule and execute tasks asynchronously in your Django application. This is particularly useful for handling background tasks like sending emails, generating reports, or performing data processing without affecting the responsiveness of your web application.
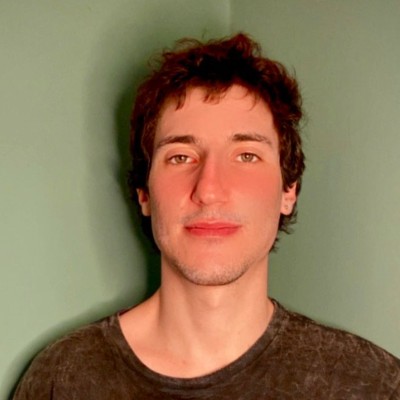
Previously at
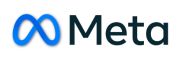
Experienced Full-stack Developer with a focus on Django, having 7 years of expertise. Worked on diverse projects, utilizing React, Python, Django, and more.