How to add search functionality to a Django Rest Framework API?
Adding search functionality to a Django Rest Framework (DRF) API can greatly enhance the usability of your application. Here’s a step-by-step guide on how to implement search in a DRF API:
- Install Required Packages:
Before you start, make sure you have DRF and any search-related packages installed. Commonly used packages for search include `django-filter` and `django-rest-framework-search`.
- Create a Serializer:
Begin by creating a serializer for the model you want to search. The serializer should define the fields you want to include in your search results. You can use DRF serializers for this purpose.
- Configure Filtering:
To enable search, use DRF’s filtering capabilities. In your API view or viewset, define the filter backend you want to use. For example, if you’re using `django-filter`, you can set `filter_backends` to include `’django_filters.rest_framework.DjangoFilterBackend’`.
- Create a Filter Class:
If you’re using `django-filter`, create a filter class that defines the fields you want to filter on. You can specify which fields are searchable, set filtering options, and define custom filter methods if needed.
- Apply Filters:
In your view or viewset, apply the filters to your queryset using the `filter_queryset` method. This filters the queryset based on the search parameters provided in the request.
- Configure URL Routing:
Set up URL routing to define the endpoints for your search functionality. You can use DRF’s routers or manually define URL patterns to map to your view or viewset.
- Implement Search Endpoint:
Create an API endpoint that accepts search queries from clients. This endpoint should use the serializer and filtering configuration you’ve set up to perform the search.
- Handling Search Queries:
In your view or viewset, access the search query from the request and apply it to the queryset. Depending on your filter class and configuration, this will filter the results based on the search criteria.
- Response Formatting:
Format the search results using your serializer and return them as JSON responses to the client.
- Testing:
Write unit tests to ensure that your search functionality works as expected. DRF provides testing tools to help with this.
- Documentation:
Update your API documentation to inform users about the search endpoint and the available search parameters.
- Pagination:
If your search results could be large, consider implementing pagination to limit the number of results returned per request.
By following these steps, you can add powerful search functionality to your DRF API, making it easier for users to find and access the data they need. Whether you’re building a simple search feature or a more advanced search system, DRF’s flexibility and extensibility make it a robust choice for implementing search in Django-based applications.
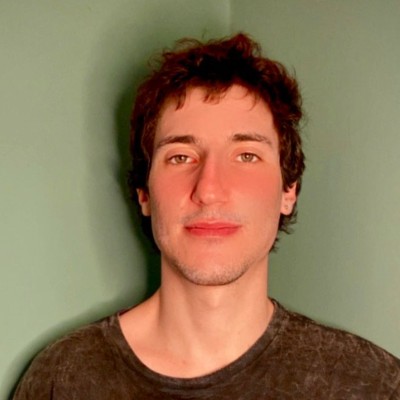
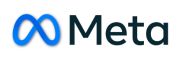