Transform Your Django App with Simple Caching Strategies
In the realm of web development, performance is king. As Django developers, we’re constantly looking for ways to make our applications run faster and smoother. One of the most effective tools in achieving this goal is server-side caching. This blog post delves into how Django leverages caching to enhance performance, providing practical examples and insights.
1. Understanding Caching
Before diving into Django-specific details, let’s understand what caching is. Caching is a technique that stores a copy of a given resource and serves it for subsequent requests. This process reduces the time to fetch data from the server or database, improving the overall response time of your application.
2. Why Caching is Crucial in Django
Django, being a high-level Python web framework, emphasizes the rapid development of clean, pragmatic design. As websites scale, the demand on the server increases. Caching provides a way to alleviate this strain, particularly in data retrieval operations, which are often the most resource-intensive part of a web application.
3. Types of Caching in Django
Django supports various types of caching:
- File-based Caching: Data is stored in the file system.
- Database Caching: Caches data in your database.
- Memory Caching: Stores data in memory, offering the fastest way to cache.
3.1. Implementing Caching in Django
Let’s look at how to implement caching in a Django project.
Example 1: Basic File-based Caching
```python CACHES = { 'default': { 'BACKEND': 'django.core.cache.backends.filebased.FileBasedCache', 'LOCATION': '/path/to/your/cache/directory', } } ```
This example shows a simple file-based caching setup. You define the cache in your `settings.py` file, specifying the backend and location.
Example 2: Database Caching
```python CACHES = { 'default': { 'BACKEND': 'django.core.cache.backends.db.DatabaseCache', 'LOCATION': 'my_cache_table', } } ```
For database caching, you specify a database table that Django will use to store cache data.
Example 3: Using Memcached
```python CACHES = { 'default': { 'BACKEND': 'django.core.cache.backends.memcached.MemcachedCache', 'LOCATION': '127.0.0.1:11211', } } ```
Memcached is a popular choice for memory caching in Django applications. It’s an in-memory key-value store that is fast and efficient.
3.2. Advanced Caching Strategies
Beyond basic setup, Django offers advanced caching strategies like per-view caching, template fragment caching, and low-level cache API for fine-grained control.
Per-View Caching
You can cache individual views using the `cache_page` decorator:
```python from django.views.decorators.cache import cache_page @cache_page(60 * 15) def my_view(request): # your view logic ```
Template Fragment Caching
Django allows caching parts of a template:
```html {% load cache %} {% cache 500 sidebar %} <!-- Expensive processing --> {% endcache %} ```
4. Best Practices and Considerations
- Choose the Right Cache Backend: The choice depends on your application’s needs. Memory caching is faster but more volatile.
- Set Appropriate Cache Expiry: Cache data should expire to prevent serving stale content.
- Cache Invalidation: Update the cache when data changes.
Conclusion
Caching is an essential aspect of Django development. Properly implemented, it can drastically improve the performance and scalability of your web applications.
Further Reading and References:
- Django’s Official Caching Documentation
- Understanding Caching in Django
- Memcached Official Documentation
- Database Caching in Django – A Detailed Guide
You can check out our other blog posts to learn more about Django. We bring you a complete guide titled Django and Accessibility: Building Inclusive Web Applications along with the Django and Scalability: Building Web Apps for High Traffic and Django Templates: Creating Dynamic and Responsive Web Pages which will help you understand and gain more insight into the Django programming language.
Table of Contents
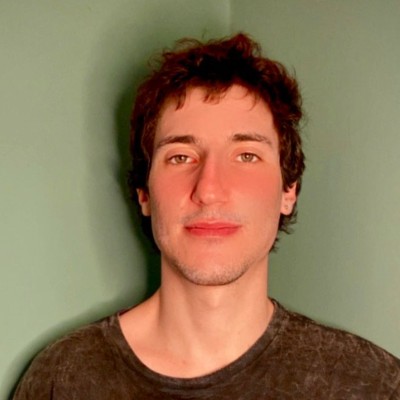
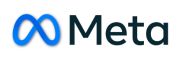