Django and Serverless Architecture: Building Serverless Web Apps
In the fast-paced world of web development, scalability and cost-efficiency are paramount. Traditional web hosting solutions often struggle to meet the demands of modern applications, leading to slow load times and high operational costs. This is where serverless architecture comes into play, offering a dynamic and efficient way to host web applications. In this guide, we’ll explore how to combine the power of Django, a popular Python web framework, with serverless architecture to build scalable and cost-effective web applications.
Table of Contents
1. Understanding Serverless Architecture
1.1. What is Serverless Architecture?
Serverless architecture is a cloud computing model that allows you to build and run applications without the need to manage the underlying infrastructure. In a serverless setup, cloud providers like AWS, Azure, or Google Cloud take care of server provisioning, scaling, and maintenance, allowing developers to focus solely on writing code. Serverless applications are event-driven and can automatically scale to handle varying workloads, making them an ideal choice for web applications with unpredictable traffic patterns.
1.2. Advantages of Serverless Architecture
- Scalability: Serverless applications can automatically scale up or down in response to incoming requests. This elasticity ensures your app can handle traffic spikes without manual intervention.
- Cost-Efficiency: With serverless, you only pay for the computing resources you actually use. This can result in significant cost savings compared to traditional hosting models.
- Reduced Operational Burden: Cloud providers handle server provisioning, maintenance, and security, reducing the operational burden on development teams.
1.3. Challenges of Serverless Architecture
While serverless architecture offers numerous benefits, it also presents some challenges:
- Cold Starts: Serverless functions can experience “cold starts,” which are delays in execution when a function is invoked for the first time. This can impact the responsiveness of your application.
- Limited Execution Time: Serverless functions typically have a maximum execution time, which can be a limitation for long-running tasks.
- Vendor Lock-In: Adopting a specific cloud provider’s serverless offering may result in vendor lock-in, making it challenging to migrate to a different platform.
2. Introducing Django
2.1. Why Choose Django?
Django is a high-level Python web framework that is renowned for its simplicity, robustness, and scalability. It follows the “batteries-included” philosophy, providing developers with a wide range of built-in tools and libraries for common web development tasks, such as URL routing, database management, and user authentication. Here are some reasons why Django is an excellent choice for building web applications:
- Rapid Development: Django’s built-in features and conventions enable developers to create web applications quickly.
- Security: Django includes security features like protection against common web vulnerabilities, making it a secure choice for application development.
- Community and Ecosystem: Django boasts a large and active community, along with a vast ecosystem of packages and extensions to enhance your application.
2.2. Django and Serverless: A Perfect Match
While Django is typically associated with traditional server-based hosting, it can also be adapted to work seamlessly with serverless architecture. The key to this synergy lies in breaking down the Django application into smaller, independent components that can be deployed as serverless functions. These functions can then be triggered by events such as HTTP requests, database updates, or file uploads.
In the following sections, we’ll explore how to build a serverless Django web app step by step.
3. Building a Serverless Django Web App
3.1. Setting Up Your Development Environment
Before you start building a serverless Django web app, you’ll need to set up your development environment. Ensure you have the following tools installed:
- Python: Django is a Python web framework, so you’ll need Python installed on your machine.
- AWS Account: You’ll need an AWS account to use AWS Lambda and other AWS services.
- Serverless Framework: The Serverless Framework simplifies the deployment of serverless applications. Install it using npm or yarn.
3.2. Creating a Django Application
To create a Django application, follow these steps:
bash # Create a virtual environment python -m venv myenv source myenv/bin/activate # Install Django pip install django # Create a new Django project django-admin startproject myproject cd myproject # Create a Django app python manage.py startapp myapp
This sets up a basic Django project structure. Now, you can define your models, views, and templates as you would in a traditional Django application.
3.3. Integrating Django with AWS Lambda
To integrate Django with AWS Lambda, you’ll need to create serverless functions that handle HTTP requests. The Serverless Framework simplifies this process. Create a serverless.yml file in your project’s root directory with the following content:
yaml service: my-django-app provider: name: aws runtime: python3.8 stage: dev region: us-east-1
This configuration specifies the service name, AWS as the provider, the Python 3.8 runtime, the development stage, and the AWS region.
Next, define your serverless functions:
yaml functions: api: handler: myapp.wsgi.handler events: - http: path: /{proxy+} method: ANY
Here, we define an api function that uses the myapp.wsgi.handler as its handler. This handler will route incoming HTTP requests to your Django application.
3.4. Storing Data with AWS DynamoDB
In a serverless architecture, it’s advisable to use serverless-compatible databases like AWS DynamoDB. To integrate DynamoDB with your Django app, you can use the boto3 library for Python. Install it using pip:
bash pip install boto3
Next, configure your Django settings to use DynamoDB as the database backend. Update your settings.py file:
python # settings.py import os import boto3 # ... # Use DynamoDB as the database backend DATABASES = { 'default': { 'ENGINE': 'django.db.backends.dynamodb', 'NAME': 'my-django-app', 'ENDPOINT': os.environ.get('DYNAMODB_ENDPOINT'), 'ACCESS_KEY': os.environ.get('AWS_ACCESS_KEY_ID'), 'SECRET_KEY': os.environ.get('AWS_SECRET_ACCESS_KEY'), 'REGION': os.environ.get('AWS_REGION', 'us-east-1'), } }
3.5. Handling Authentication and Authorization
Security is paramount in web applications. You can leverage AWS Cognito, Amazon’s identity management service, to handle user authentication and authorization. Configure Cognito in your serverless.yml:
yaml # serverless.yml resources: Resources: CognitoUserPool: Type: AWS::Cognito::UserPool Properties: UserPoolName: my-django-app UsernameAttributes: - email AutoVerifiedAttributes: - email CognitoUserPoolClient: Type: AWS::Cognito::UserPoolClient Properties: ClientName: my-django-app-client UserPoolId: Ref: CognitoUserPool
Now, you can integrate Cognito with your Django application to handle user authentication.
3.6. Deploying Your Serverless Django App
Deploying your serverless Django app is straightforward with the Serverless Framework. Run the following commands:
bash # Deploy your serverless app serverless deploy # Deploy the Django app to AWS Lambda sls wsgi deploy
This will package and deploy your application to AWS Lambda, making it accessible via API Gateway.
3.7. Monitoring and Scaling
One of the advantages of serverless architecture is the ability to monitor and scale your application easily. AWS provides tools like CloudWatch for monitoring and AWS Auto Scaling for automatic scaling based on traffic.
4. Best Practices for Serverless Django
4.1. Optimize Your Code
To make the most of serverless architecture, optimize your code for efficiency. Minimize dependencies, use smaller package sizes, and consider using serverless-compatible libraries.
4.2. Secure Your Serverless Django App
Security remains a top concern. Implement proper authentication, authorization, and data encryption to protect your application and user data.
4.3. Cost Management
While serverless can be cost-effective, it’s crucial to monitor your usage to avoid unexpected bills. Set up billing alerts and regularly review your resources for optimization.
Conclusion
Django and serverless architecture can work hand in hand to create scalable, cost-effective web applications. By breaking down your Django app into serverless functions and leveraging AWS services, you can build web apps that automatically scale with your needs while minimizing operational overhead. Keep exploring and experimenting with this powerful combination to unlock new possibilities in web development. Happy coding!
In this comprehensive guide, we’ve covered the fundamentals of serverless architecture, introduced Django as a robust web framework, and provided a step-by-step approach to building a serverless Django web app. We’ve also discussed best practices for optimizing your serverless Django application and managing costs effectively. Now, you’re well-equipped to embark on your journey to building serverless web apps with Django.
Table of Contents
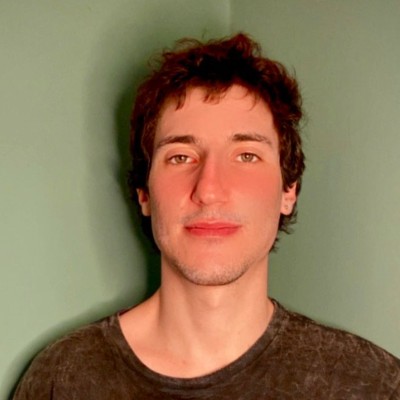
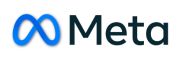