Master the Art of Streamlining Development with Django and Continuous Integration
Continuous Integration (CI) is an essential component of modern software development. In its essence, CI is a practice that encourages developers to integrate their code into a shared repository frequently. Each integration is then verified by an automated build and automated tests.
Table of Contents
When we talk about web development, Django, a high-level Python Web framework, is a popular choice among developers. It allows for rapid development, clean, pragmatic design, and encourages the Don’t Repeat Yourself (DRY) philosophy. But how do we combine Django and Continuous Integration to streamline the development workflow?
In this blog post, we’ll dive deep into how to integrate Django with a CI system. We will use GitHub Actions as our CI tool for this article, but the principles are widely applicable to other CI systems as well.
1. Why Continuous Integration?
Before we jump into the nitty-gritty details, let’s take a moment to discuss why you should consider implementing Continuous Integration into your development workflow.
CI brings numerous benefits to the development process:
– Early bug detection: Since CI requires frequent code integrations, issues are detected and addressed early, reducing the overall cost of fixing them.
– Code quality: CI often involves running code quality checks and static code analysis tools, which help improve the quality of the code.
– Streamlined testing: Automated tests are an integral part of CI, which means that your code is always tested, ensuring that any new changes don’t break existing functionality.
– Faster release cycles: Since integration issues are dealt with on an ongoing basis, it’s easier and quicker to release new versions of the software.
Now, let’s dive into how to use Django with Continuous Integration.
2. Setting up a Django Project
First, we need a Django project. For the sake of brevity, we won’t cover how to set up a Django project from scratch, but Django’s official documentation provides a great starting point.
Suppose you already have a Django project in a GitHub repository. Your project should have a structure similar to this:
``` your-django-project/ ??? manage.py ??? your_django_app/ ? ??? __init__.py ? ??? settings.py ? ??? urls.py ? ??? wsgi.py ??? requirements.txt ```
Next, ensure that your Django application has a set of working tests. Django supports writing tests out of the box, and you can verify that your tests are working locally by running `python manage.py test` in your Django project’s root directory.
3. Setting up GitHub Actions
With your Django project and tests ready, we can proceed to set up GitHub Actions for our repository. In your project root, create a new directory named `.github` and a subdirectory named `workflows`. In the `workflows` directory, create a new file named `django.yml`.
Your project structure should look like this now:
``` your-django-project/ ??? .github/ ? ??? workflows/ ? ? ??? django.yml ??? manage.py ??? your_django_app/ ? ??? __init__.py ? ??? settings.py ? ??? urls.py ? ??? wsgi.py ??? requirements.txt ```
Open the `django.yml` file and start defining your GitHub Actions workflow. Here’s a sample workflow configuration:
```yaml name: Django CI on: push: branches: [ main ] pull_request: branches: [ main ] jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Set up Python 3.8 uses: actions/setup-python@v2 with: python-version: 3.8 - name: Install dependencies run: | python -m pip install --upgrade pip pip install -r requirements.txt - name: Run tests run: | python manage.py test ```
This workflow runs every time someone pushes to the `main` branch or opens a pull request against it. The job will run on an Ubuntu machine and perform the following steps:
– Check out the repository code.
– Set up a Python 3.8 environment.
– Install the project dependencies defined in `requirements.txt`.
– Run the Django tests with `python manage.py test`.
This is a simple workflow that can be extended as per your project’s needs. For example, you can add steps to run linters like flake8, code formatters like black, or security checks like bandit.
4. Continuous Integration in Action
Now that we’ve set up our GitHub Actions workflow, let’s see it in action.
When you push your code to the `main` branch or open a pull request against it, GitHub automatically triggers the CI workflow. You can see the progress and results of the workflow in the “Actions” tab of your GitHub repository.
If all the tests pass and the workflow completes successfully, you’ll see a green checkmark next to the commit in GitHub, indicating that your changes are safe to merge. If there’s a failure, you can inspect the logs to figure out what went wrong, fix it, and push again.
This continuous feedback loop can help you catch and fix integration issues early and continuously deliver quality software.
Final Thoughts
We’ve seen how Django and Continuous Integration, using GitHub Actions, can be used together to streamline your development workflow. This setup allows you to catch errors and integration issues early, improve code quality, and deliver software faster. It further underlines the value when you hire Django developers, given their proficiency with these tools and practices.
It’s important to note that while this example uses Django and GitHub Actions, the principles of Continuous Integration are the same regardless of the specific technologies used. Whether you’re using Flask or Node.js, GitLab or Bitbucket, you can—and should—use Continuous Integration to streamline your workflow and improve your software delivery process.
Continuous Integration is more than just a tool or a practice. It’s a philosophy that, when fully embraced, can lead to significant improvements in team collaboration, software quality, and development speed. This is especially notable when you hire Django developers, as they are adept at integrating these practices into their work. It should be a critical part of every modern software development team’s toolkit.
Table of Contents
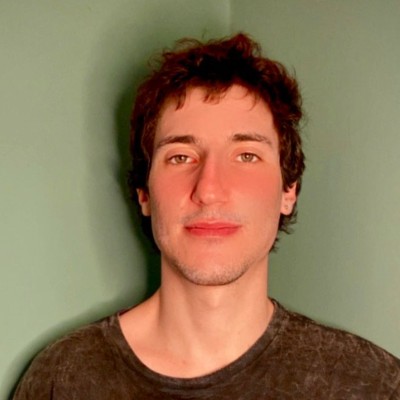
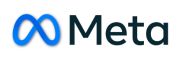