Quality Assurance in Django: A Step-by-Step Guide to Testing Your Code
Software testing is an essential phase in the software development process, critical to ensuring the reliability and quality of the application. Whether you’re coding yourself or planning to hire Django developers, understanding how to identify and fix bugs or discrepancies early in the process significantly reduces the overall cost and time required for maintenance. In Django, the testing framework is a built-in feature, providing a set of tools to build and execute tests. This advantage can be leveraged whether you’re developing in-house or if you decide to hire Django developers. In this blog post, we’ll explore various testing methods in Django, offering practical examples to enhance the understanding of this vital topic.
Why Django Testing is Important
Before diving into the technicalities, let’s clarify why testing is so critical in Django. In a nutshell, Django testing:
- Ensures Code Quality: Testing ensures that your application behaves as expected. It validates the functionalities against specified requirements and ensures adherence to coding standards.
- Detects and Mitigates Bugs: Testing can expose issues that might be hidden during the development phase, allowing developers to rectify them early on.
- Facilitates Refactoring: Test cases make the refactoring process smoother. They provide a safety net and allow developers to make changes without worrying about breaking the existing functionalities.
- Speeds Up Integration: Tests allow developers to confidently integrate components or modules without causing a ripple effect of bugs.
Types of Django Testing
Django supports several types of tests, including unit tests, integration tests, and functional or end-to-end tests.
– Unit Testing checks the functionality of individual components in isolation.
– Integration Testing checks how different components interact and work together.
– Functional Testing checks if the application meets all the specified requirements and functions properly.
Let’s understand these in detail using examples.
Setting Up Your Testing Environment
Before we begin, let’s set up our testing environment. Django comes with a built-in testing client that lets you simulate GET and POST requests and observe how your views and templates respond. Ensure that you have Django’s testing framework set up correctly in your project’s settings:
```python INSTALLED_APPS = [ # ... 'django.contrib.staticfiles', # Required for serving static files during tests # ... 'django.contrib.admin', # ... ] TEST_RUNNER = 'django.test.runner.DiscoverRunner' ```
Now, let’s write some tests.
Unit Testing
Suppose we have a Django app called ‘Blog’ with a model ‘Post’. Let’s create a unit test for the ‘Post’ model.
```python from django.test import TestCase from .models import Post class PostModelTest(TestCase): def setUp(self): Post.objects.create(title='Test Title', content='Test Content') def test_post_content(self): post = Post.objects.get(id=1) expected_object_name = f'{post.title}' expected_object_content = f'{post.content}' self.assertEqual(expected_object_name, 'Test Title') self.assertEqual(expected_object_content, 'Test Content') ```
In this example, the `setUp()` method is used to set up the database state for the test. Then, we’re checking the content of the post, using the `assertEqual()` method.
Integration Testing
In integration testing, we test how different components of our application interact with each other. Let’s say we have a view in our ‘Blog’ app that lists all posts:
```python from django.views.generic import ListView from .models import Post class PostListView(ListView): model = Post template_name = 'blog/home.html' context_object_name = 'posts' ```
Now, let’s write an integration test for this view:
```python from django.test import TestCase from django.urls import reverse from .models import Post class PostListViewTest(TestCase): def setUp(self): Post.objects.create(title='Test Title', content='Test Content') def test_view_url_exists_at_desired_location(self): resp = self.client.get('/blog/') self.assertEqual(resp.status_code, 200) def test_view_url_accessible_by_name(self): resp = self.client.get(reverse('blog-home')) self.assertEqual(resp.status_code, 200) def test_view_uses_correct_template(self): resp = self.client.get(reverse('blog-home')) self.assertTemplateUsed(resp, 'blog/home.html') ```
Here, we’re checking if the URL exists, if it’s accessible by name, and if it uses the correct template.
Functional Testing
Functional tests involve testing the application against user interactions. Suppose you have a form in your ‘Blog’ app that allows users to add new posts. Here’s how a functional test might look like:
```python from django.test import TestCase from django.urls import reverse from .models import Post class NewPostTest(TestCase): def test_new_post_form(self): response = self.client.post(reverse('post_new'), { 'title': 'New Title', 'content': 'New content', }) self.assertEqual(response.status_code, 200) self.assertContains(response, 'New Title') self.assertContains(response, 'New content') def test_new_post_form_invalid(self): response = self.client.post(reverse('post_new'), { 'title': '', 'content': '', }) self.assertEqual(response.status_code, 200) self.assertContains(response, 'This field is required.') ```
In this test, we’re checking the scenario where a user enters valid data to create a post and also when a user enters invalid data.
Running the Tests
Once the tests are defined, we can execute them using Django’s test runner. The command to run the tests is simple:
``` python manage.py test ```
This command will look for tests in any file named with the pattern `test*.py` under the current working directory.
Conclusion
Testing is an indispensable part of software development. It ensures the quality, reliability, and performance of your Django application. Whether you’re a novice just starting your Django journey or a pro consider hiring Django developers, a thorough understanding of comprehensive testing – from unit to integration and functional tests – can significantly enhance the end-user experience, improve the maintainability of your codebase, and increase overall project success. Integrating testing into your Django development routine is not just a recommended practice, it’s a winning strategy for high-quality and reliable coding. Happy coding!
Table of Contents
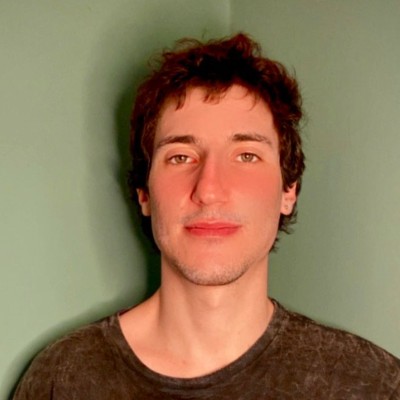
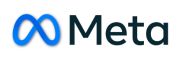