Twilio & Django: The Ultimate Combo for Next-Level Communication Apps
Django, the popular web framework built on Python, has proven to be versatile and efficient for many web applications. Twilio, on the other hand, offers cloud communications services, enabling you to add voice, SMS, and more to your apps. Combining the power of both can lead to some impressive results.
Table of Contents
In this post, we’ll explore how you can integrate Django with Twilio to build voice and SMS-based applications, complete with examples!
1. Setting Up Your Environment
Before we begin, ensure you have the following installed:
– Python
– Django
– Twilio Python SDK (`pip install twilio`)
You’d also need a Twilio account and your `ACCOUNT_SID`, `AUTH_TOKEN`, and a Twilio phone number. These are essential for sending and receiving messages and calls.
2. Building a Simple SMS Application with Django and Twilio
2.1. Setting Up Django:
If you haven’t already, set up a Django project:
```bash django-admin startproject sms_project cd sms_project ```
2.2. Create a new app:
```bash python manage.py startapp sms_app ```
2.3. Add your app to `INSTALLED_APPS` in `settings.py`:
```python INSTALLED_APPS = [ # ... 'sms_app', ] ```
2.4. Setting Up Twilio in your Django app:
In `sms_app/views.py`:
```python from django.http import HttpResponse from twilio.rest import Client def send_sms(request): account_sid = 'YOUR_ACCOUNT_SID' auth_token = 'YOUR_AUTH_TOKEN' client = Client(account_sid, auth_token) message = client.messages.create( body='Hello from Django!', from_='+1234567890', # Your Twilio number to='+0987654321' # Your phone number ) return HttpResponse(f"Message SID: {message.sid}") ```
This view will send an SMS when accessed.
2.5. Setting up the URL
In `sms_project/urls.py`:
```python from django.urls import path from sms_app.views import send_sms urlpatterns = [ path('send-sms/', send_sms, name='send_sms'), ] ```
Now, running your Django server and visiting `http://127.0.0.1:8000/send-sms/` will send an SMS to the specified number.
3. Building a Voice Application with Django and Twilio
Let’s build a simple application that reads out a message when a user calls your Twilio number.
3.1. Set up a new view in `sms_app/views.py`:
```python from django.http import HttpResponse from twilio.twiml.voice_response import VoiceResponse def answer_call(request): response = VoiceResponse() response.say('Hello! You have reached the Django and Twilio voice application.', voice='alice') return HttpResponse(str(response), content_type='text/xml') ```
3.2. Add the new URL in `sms_project/urls.py`:
```python from sms_app.views import answer_call urlpatterns = [ #... path('answer-call/', answer_call, name='answer_call'), ] ```
3.3. Configure Twilio:
Go to your [Twilio Dashboard](https://www.twilio.com/console), and navigate to your phone numbers. For your Twilio number, set the voice webhook to `http://yourdomain.com/answer-call/`.
Now, whenever someone calls your Twilio number, they’ll hear the message from your Django application!
4. Tips and Advanced Integration
- Storing Credentials Securely: Avoid hardcoding your `ACCOUNT_SID` and `AUTH_TOKEN`. Use Django’s `secrets` module or environment variables.
- Handling Incoming SMS: By setting an SMS webhook in your Twilio dashboard, you can handle incoming messages just as easily as outgoing ones.
- Django Signals: Use Django signals to trigger SMS or voice calls based on database events.
Conclusion
Integrating Django and Twilio can lead to some powerful applications, from simple notifications to complex voice-driven systems. By using the Twilio Python SDK and Django’s robust framework, developers can easily craft intuitive and interactive communication solutions. Dive in and explore the vast potential!
Table of Contents
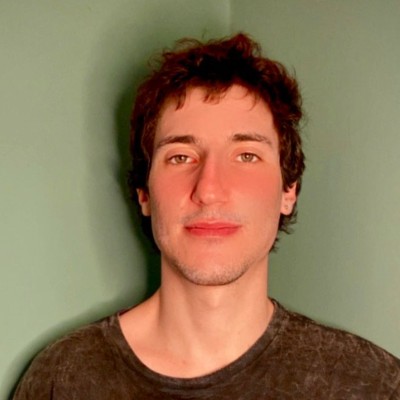
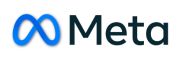