How to add user authentication to Django REST framework API using JWT (JSON Web Tokens)?
Adding user authentication to a Django REST framework (DRF) API using JSON Web Tokens (JWT) is a popular and secure approach. JWTs allow you to verify the identity of users without storing sessions on the server, making them suitable for stateless APIs. Here’s how you can implement JWT-based authentication in a Django REST framework API:
- Install Required Packages:
Start by installing the necessary packages:
```bash pip install djangorestframework djangorestframework-jwt ```
- Configure Django Settings:
In your Django project’s settings, add the following configurations:
```python # settings.py REST_FRAMEWORK = { 'DEFAULT_AUTHENTICATION_CLASSES': ( 'rest_framework_jwt.authentication.JSONWebTokenAuthentication', ), } # JWT settings from datetime import timedelta JWT_AUTH = { 'JWT_EXPIRATION_DELTA': timedelta(days=1), } ```
Adjust the `JWT_EXPIRATION_DELTA` to set the token’s expiration time as needed.
- Create API Views:
Create your API views as usual and apply the `@authentication_classes` and `@permission_classes` decorators to secure them:
```python from rest_framework.decorators import authentication_classes, permission_classes from rest_framework.permissions import IsAuthenticated from rest_framework.response import Response from rest_framework.views import APIView from rest_framework_jwt.authentication import JSONWebTokenAuthentication @authentication_classes([JSONWebTokenAuthentication]) @permission_classes([IsAuthenticated]) class YourSecuredView(APIView): def get(self, request): return Response({'message': 'This is a secured view.'}) ```
In this example, the `YourSecuredView` is protected, and users must be authenticated to access it.
- Obtain JWT Tokens:
To obtain a JWT token, users typically need to send a POST request to a login endpoint with their credentials. DRF provides built-in views for this, such as `obtain_jwt_token` and `refresh_jwt_token`. You can configure URLs and use these views in your project.
- Include JWT Token in Requests:
Once users obtain a token, they should include it in the `Authorization` header of subsequent API requests as `Bearer <token>`.
With these steps, you can add JWT-based user authentication to your Django REST framework API. It provides a secure and scalable way to protect your endpoints and ensure that only authorized users can access them.
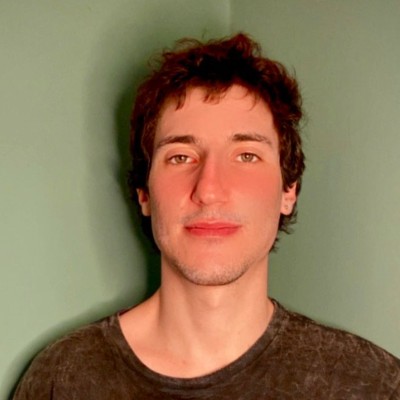
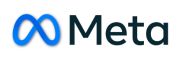