How to implement user authorization and access control in Django
Implementing user authorization and access control in Django is essential for managing who can perform certain actions and access specific parts of your web application. Django provides a robust built-in authentication and authorization system that makes this process straightforward.
Here’s a step-by-step guide on how to implement user authorization and access control in Django:
- User Authentication:
Start by implementing user authentication. Django’s authentication system allows users to create accounts, log in, and log out. You can use the built-in `User` model or extend it to add custom user data.
- User Groups and Permissions:
Django provides a flexible system for managing user permissions. Define permissions at the model level using the `Meta` class or add custom permissions. Create user groups and assign permissions to these groups. For example, you can create groups like “Admins,” “Editors,” and “Viewers.”
- Decorators for Access Control:
Django offers decorators like `@login_required` and `@permission_required` that you can use to restrict access to specific views based on user authentication and permissions. For example, to restrict access to a view to users with a specific permission:
```python from django.contrib.auth.decorators import permission_required @permission_required('your_app.can_do_something') def your_view(request): # Your view logic here ```
- Object-Level Permissions:
For fine-grained control over object-level permissions, you can implement custom logic in your views or use Django’s built-in `django.contrib.auth.mixins.PermissionRequiredMixin` in class-based views.
- Template Tags and Filters:
Use Django’s template tags and filters to control the visibility of elements in your templates based on user permissions. For instance, you can hide or show buttons or menu items depending on the user’s role.
- Custom Authorization Logic:
If you require more complex authorization logic, you can implement custom authorization backends or middleware to tailor the access control process to your application’s specific needs.
By following these steps and leveraging Django’s built-in authentication and authorization system, you can implement user authorization and access control in your Django application efficiently and securely. This allows you to manage user permissions, restrict access to views, and control what users can do within your web application.
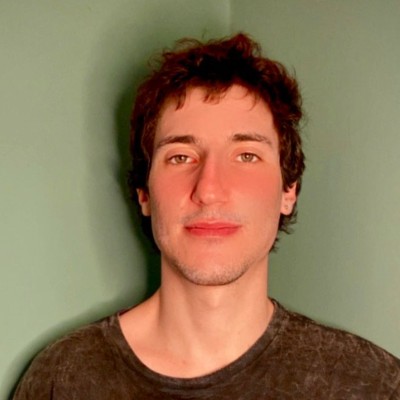
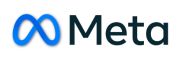