Version Control Simplified: Git Best Practices for Your Django Projects
Version control is an essential aspect of software development. With complex frameworks like Django, where multiple files change and interplay with each other, version control becomes even more critical. One of the most popular version control systems today is Git, and in this post, we’ll explore how to manage a Django project with Git.
Table of Contents
Django, a robust and popular web framework written in Python, offers developers a platform to build scalable and maintainable web applications. But like every software project, Django applications evolve, which requires a reliable version control system. That’s where Git comes in handy.
1. Getting Started with Git in Your Django Project
Before you can manage your codebase, you need to initialize Git in your Django project:
- Navigate to the root of your Django project using the terminal or command prompt.
- Type `git init`. This initializes a new Git repository and begins tracking an existing directory.
2. Best Practices: What to Include and Exclude
Django projects have specific files that shouldn’t be included in version control, like `db.sqlite3` or the `__pycache__` directories. Thankfully, Git allows you to use a `.gitignore` file to specify patterns of files that it should overlook.
Example `.gitignore` for Django:
``` *.log *.pot *.pyc *.pyo *.db *.sqlite3 *.sqlite-journal __pycache__/ *.egg-info/ *.egg media/ ```
This setup excludes cache files, logs, databases, and the media directory, among other things.
3. Committing Changes
As you make changes to your Django project, it’s crucial to periodically commit these changes. A commit serves as a checkpoint, allowing you to save the current state of your project.
Example:
You just added a new model to your `models.py`:
```python class Blog(models.Model): title = models.CharField(max_length=200) content = models.TextField() date_posted = models.DateTimeField(auto_now_add=True) ```
Commit this change by:
- Adding the files to staging: `git add .`
- Committing with a meaningful message: `git commit -m “Added Blog model to models.py”`
4. Branching Out
When developing new features or testing something out, you don’t want to mess up your main codebase. This is where branching becomes crucial.
Example:
You want to add a new feature called “comments” to your blog.
- Create a new branch: `git checkout -b feature-comments`
- Now you’re on the new branch, make your changes, and commit them as you go.
- Once done, you can merge this branch back into the main branch or continue developing.
5. Merging and Handling Conflicts
When merging branches, there’s a possibility of conflicts, especially if the same part of a file was edited differently in both branches.
Example:
Both in `main` and `feature-comments` branches, the same line in `views.py` was changed. Git will notify you of a conflict.
To resolve:
- Open the conflicting file. Git marks the problematic area as:
``` <<<<<<< HEAD ...code from the main branch... ======= ...code from the feature-comments branch... >>>>>>> feature-comments ```
- Edit the file to how you want the final version to look, and then save it.
- Commit the merged changes: `git commit -m “Resolved merge conflict in views.py”`
6. Pushing and Pulling: Syncing with Remote Repositories
It’s a good practice to store your codebase in a remote repository, such as GitHub or GitLab.
- To push your local repository to a remote, first, add the remote repository: `git remote add origin [URL to your remote repo]`
- Then, push your code: `git push -u origin main`
Whenever you want to fetch the latest changes from the remote repository, simply use: `git pull origin main`
7. Cloning a Django Project
When collaborating or working across different machines, you’ll often need to get a copy of a Django project. This is achieved via the `git clone` command.
Example:
`git clone [URL to the Django repo]`
After cloning, don’t forget to set up a virtual environment and install the required dependencies, often listed in a `requirements.txt` file.
Conclusion
Git offers an efficient way to manage and track changes in your Django projects. By using version control, you ensure that your development process remains organized, streamlined, and free from many common pitfalls. Whether you’re working alone or collaborating in a team, understanding the basics of Git and how it intertwines with Django is crucial for modern web development.
Remember, practice makes perfect. The more you use Git with Django, the more intuitive and seamless the process becomes. Happy coding!
Table of Contents
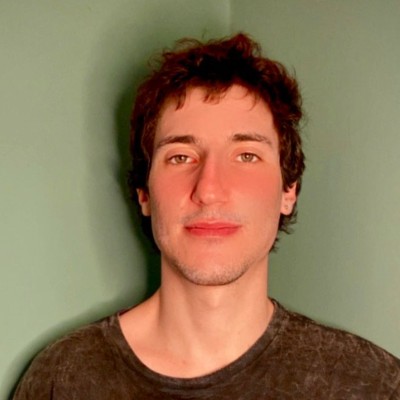
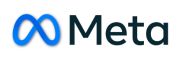