Web Scraping Simplified: Django Techniques for Efficient Data Harvesting
In the evolving world of data-driven decision-making, web scraping has emerged as a vital technique for extracting valuable information from the vast expanse of the internet. This blog post delves into how Django, a high-level Python web framework, can be harnessed to build efficient and robust web scraping tools. You can hire Django Developers for your projects to ensure greater success.
Table of Contents
1. Introduction to Web Scraping and Django
Web Scraping is the process of extracting data from websites. This data extraction is often used for data analysis, competitive intelligence, and automated testing.
Django, on the other hand, is a Python framework known for its simplicity and efficiency in building web applications. It follows the DRY (Don’t Repeat Yourself) principle, making it an excellent choice for rapid development.
2. Why Django for Web Scraping?
- Robust Framework: Django offers a well-structured environment that is ideal for both simple and complex web scraping tasks.
- Scalability: With Django, scaling up your web scraping solution is straightforward, ensuring it can handle increased loads.
- Community and Support: Django has a large community, making it easier to find solutions and examples for web scraping challenges.
3. Setting Up Your Django Environment
Before diving into scraping, ensure you have Django installed. You can follow the official Django documentation for installation instructions.
4. Building a Simple Web Scraper with Django
Step 1: Creating a Django Project
Start by creating a new Django project:
```bash django-admin startproject scraper_project cd scraper_project ```
Step 2: Setting Up a Scraper App
Create a new app within your project:
```bash python manage.py startapp scraper ```
Step 3: Writing Your Scraper
We’ll use `BeautifulSoup` and `requests` for scraping. Ensure you have these installed:
```bash pip install beautifulsoup4 requests ```
Now, let’s write a simple scraper in `views.py` of our scraper app:
```python import requests from bs4 import BeautifulSoup def scrape_website(url): response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') # Example: Extracting all headings headings = soup.find_all('h1') return [h.text for h in headings] ```
Step 4: Integrating with Django Views
In `views.py`, create a view to call our `scrape_website` function:
```python from django.http import JsonResponse def scrape_view(request): url = 'https://example.com' data = scrape_website(url) return JsonResponse({'headings': data}) ```
Step 5: Setting Up URLs
In your app’s `urls.py`, set up a URL for your scraping view:
```python from django.urls import path from .views import scrape_view urlpatterns = [ path('scrape/', scrape_view, name='scrape'), ] ```
Step 6: Running the Scraper
Run your Django server:
```bash python manage.py runserver ```
Visit `http://localhost:8000/scrape/` to see your scraper in action.
5. Advanced Techniques
5.1. Asynchronous Scraping
For more efficiency, especially with multiple requests, consider using asynchronous requests. Libraries like `aiohttp` can be useful. Learn more about asynchronous programming in Python here..
5.2. Database Integration
Storing your scraped data can be efficiently done using Django’s ORM. Define models according to the data structure you need. Read more about Django’s database models here.
6. Avoiding IP Bans and Captchas
To avoid IP bans and captchas, consider using rotating proxy services and user-agent rotation. This guide on proxy rotation could be a starting point.
7. Legal and Ethical Considerations
Always respect the `robots.txt` of websites and understand the legal implications of web scraping. The Electronic Frontier Foundation provides resources on digital privacy laws.
Conclusion
Django, with its robust framework, scalability, and supportive community, proves to be an excellent choice for building web scraping tools. Whether you are scraping for data analysis, competitive intelligence, or automated testing, Django provides the tools and flexibility needed to create efficient and reliable data extraction tools.
Remember, web scraping comes with its own set of legal and ethical considerations. It’s always important to respect the privacy and terms of use of the websites you scrape.
Explore further with Django’s comprehensive documentation and join the vibrant community for more insights and support.
You can check out our other blog posts to learn more about Django. We bring you a complete guide titled Elevate Your Django Projects with Web Scraping Techniques along with the Master Real-Time Communication in Django Apps with WebSockets and The Essential Guide to Social Logins in Django with OAuth and OpenID which will help you understand and gain more insight into the Django programming language.
Table of Contents
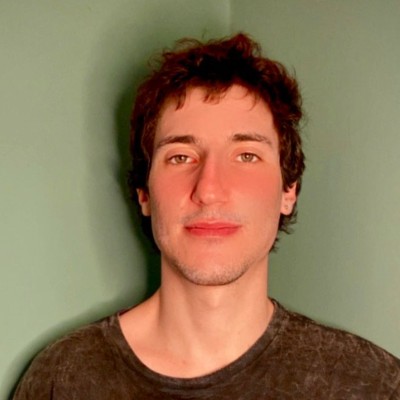
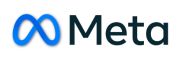