Master Real-Time Communication in Django Apps with WebSockets
The world is now moving at a pace where real-time communication is an expected standard. Users need to see what’s happening now, and not on their next refresh. This is where WebSockets come in – to provide real-time, bidirectional communication between the server and the client. And Django, one of the most popular Python frameworks, fits perfectly into this equation. But to fully capitalize on this powerful duo, you may want to consider hiring Django developers.
Table of Contents
In this blog post, we are going to explore how to integrate Django with WebSockets for real-time communication in your web application. This might seem complex initially, but with the expertise of dedicated Django developers, this integration can be a seamless process. When you hire Django developers, you can be assured of their experience in implementing cutting-edge solutions and keeping your application up-to-date with the evolving digital world.
1. Understanding WebSockets
WebSockets is a protocol providing full-duplex communication channels over a single TCP connection. It allows servers to push updates to clients in real time, thereby removing the need for periodic polling.
Unlike HTTP, where the client always initiates a connection, WebSocket provides a persistent connection between a client and a server that both parties can use to start sending data at any time.
2. Django and Channels
Historically, Django wasn’t designed to work with WebSockets. It was built to handle HTTP requests and provide HTTP responses. However, with the development of a package named Django Channels, we can now handle WebSockets and HTTP equally well.
Django Channels is essentially a project that takes Django and extends its abilities beyond HTTP – to handle WebSockets, chat protocols, IoT protocols, and more. It takes Django and turns it into a system that is capable of handling not only HTTP, but also protocols that require long-term connections like WebSockets.
3. Installing Channels
First, we need to install Channels in our Django project. You can install it via pip:
```python pip install channels ```
After the successful installation of Channels, add it to your Django project’s settings:
```python # settings.py INSTALLED_APPS = [ ... 'channels', ] ```
Also, change your Django project’s default ASGI application to the Channels routing application in the same `settings.py` file:
```python # settings.py ASGI_APPLICATION = 'your_project.routing.application' ```
This tells Channels what settings to use for your ASGI application.
4. Creating the Consumer
A consumer is a Python class where WebSocket code lives. When a user connects to the server through a WebSocket, a consumer is created and handles further communication.
Let’s create a simple consumer that accepts WebSocket connections, receives messages from the WebSocket, and sends messages to the WebSocket. We’ll use synchronous WebSocket consumer as an example:
```python # consumers.py from channels.generic.websocket import WebsocketConsumer import json class ChatConsumer(WebsocketConsumer): def connect(self): self.accept() def disconnect(self, close_code): pass def receive(self, text_data): text_data_json = json.loads(text_data) message = text_data_json['message'] self.send(text_data=json.dumps({ 'message': message })) ```
This consumer uses the `connect` method to accept the WebSocket connection. The `disconnect` method is used when the WebSocket closes. The `receive` method is called whenever a message is received from the WebSocket.
5. Routing WebSockets to the Consumer
Now we need to create a routing configuration for the WebSocket. This is a Python dictionary that maps route names to consumer objects.
```python # routing.py from django.urls import re_path from . import consumers websocket_urlpatterns = [ re_path(r'ws/chat/$', consumers.ChatConsumer.as_asgi()), ] ```
This maps `ws/chat/` to our `ChatConsumer`.
5. Wrapping Up with Frontend
The backend setup is now complete. Let’s switch to the frontend to establish a WebSocket connection.
```javascript // connect to the WebSocket var chatSocket = new WebSocket('ws://localhost:8000/ws/chat/'); // when a message is received chatSocket.onmessage = function(e) { var data = JSON.parse(e.data); console.log(data.message); }; // when the connection is closed chatSocket.onclose = function(e) { console.error('Chat socket closed unexpectedly'); }; // send a message chatSocket.send(JSON.stringify({ 'message': 'Hello, World!' })); ```
Conclusion
Real-time communication is an essential part of modern web applications. It allows users to engage with the application interactively and receive immediate updates. Django, combined with Channels, provides an effective way to manage WebSocket connections and enable real-time communication.
In this article, we have demonstrated the basic usage of Django Channels for WebSocket communication. However, in real-world applications, you might need more advanced features like channel layers for group communication, authentication, or connecting the Channels app with Django models.
Hire Django developers who can leverage the full potential of Django and Channels to enhance your web applications with real-time features, offering a seamless user experience and a high level of interactivity. Whether you need a complex real-time application or just want to improve the responsiveness of your existing app, dedicated Django developers can help you achieve your goals efficiently.
Table of Contents
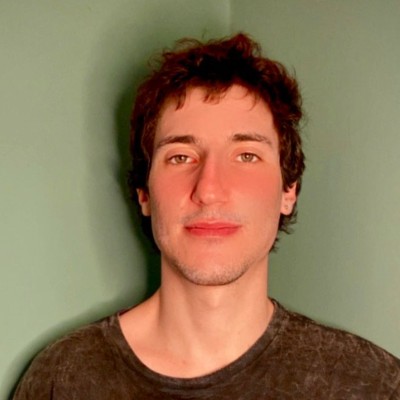
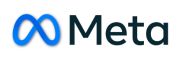