Exploring Elixir’s Behaviours: Code Reuse and Composition
Understanding Elixir’s Behaviours
Elixir’s Behaviours are a powerful abstraction mechanism that allows developers to define a shared set of functions that modules must implement. By enforcing a consistent interface across different modules, Behaviours promote code reuse, modular design, and easier testing.
Why Use Behaviours?
Using Behaviours in Elixir helps developers create clean, maintainable, and scalable code by:
– Encouraging Reusability: Behaviours allow you to define generic interfaces that can be implemented by different modules, reducing code duplication.
– Facilitating Composition: By breaking down functionality into smaller, composable modules, Behaviours promote modular design.
– Enhancing Testability: Consistent interfaces across modules make it easier to write and maintain test cases.
Implementing a Behaviour in Elixir
To define and use a Behaviour, you first declare the Behaviour with a `@callback` directive, then implement it in modules. Below is a simple example to illustrate this.
Example: Defining and Implementing a Behaviour
```elixir defmodule LoggerBehaviour do @callback log(String.t()) :: :ok end defmodule ConsoleLogger do @behaviour LoggerBehaviour @impl LoggerBehaviour def log(message) do IO.puts("Console: #{message}") end end defmodule FileLogger do @behaviour LoggerBehaviour @impl LoggerBehaviour def log(message) do File.write!("log.txt", message <> "\n", [:append]) end end ```
In this example:
– `LoggerBehaviour` defines a generic `log/1` function.
– `ConsoleLogger` and `FileLogger` implement this behaviour with their own specific logic for logging messages.
Leveraging Behaviours for Code Reuse
Behaviours are particularly useful when you have multiple modules that perform similar tasks but with different implementations. By defining a Behaviour, you can ensure each module adheres to a consistent interface, making it easier to swap implementations.
Example: Using Behaviour for Multiple Implementations
```elixir defmodule Application do def run(logger) do logger.log("Starting application...") end end Application.run(ConsoleLogger) Application.run(FileLogger) ```
Here, the `Application.run/1` function can accept any module that implements the `LoggerBehaviour`, promoting flexibility and reuse.
Composition with Behaviours
Elixir’s Behaviours also support composition by enabling developers to break down complex functionality into smaller, reusable modules.
Example: Composing Functionality with Behaviours
```elixir defmodule TimestampedLogger do @behaviour LoggerBehaviour @impl LoggerBehaviour def log(message) do timestamp = :os.system_time(:seconds) message_with_timestamp = "[#{timestamp}] #{message}" ConsoleLogger.log(message_with_timestamp) end end ```
In this example:
– `TimestampedLogger` composes functionality by adding a timestamp to the log message before passing it to `ConsoleLogger`.
Testing Behaviour Implementations
Testing is more straightforward when using Behaviours because you can write tests against the Behaviour’s interface rather than individual module implementations.
Example: Testing a Behaviour Implementation
```elixir defmodule ConsoleLoggerTest do use ExUnit.Case test "logs message to console" do assert capture_io(fn -> ConsoleLogger.log("Test message") end) == "Console: Test message\n" end end ```
Here, the test ensures that `ConsoleLogger` correctly implements the `log/1` function defined in `LoggerBehaviour`.
Conclusion
Elixir’s Behaviours offer a robust way to enforce consistent interfaces, promote code reuse, and enable composition. By leveraging Behaviours, you can write more maintainable, flexible, and testable Elixir code. Whether you’re developing logging utilities, APIs, or complex systems, understanding and using Behaviours will significantly enhance your Elixir projects.
Further Reading:
Table of Contents
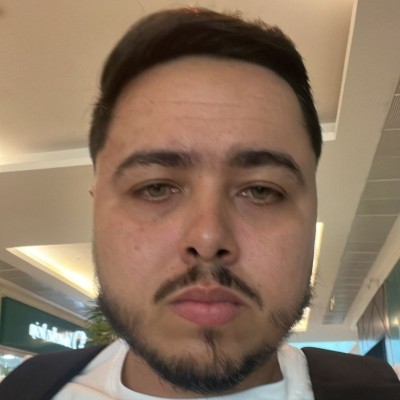
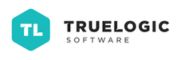