Elixir and Blockchain: Building Decentralized Applications
Blockchain technology has revolutionized various industries by providing secure, transparent, and decentralized solutions. Elixir, a functional programming language built on the Erlang VM, is well-suited for developing scalable and reliable decentralized applications (dApps). This article explores how Elixir can be used to build blockchain-based applications, highlighting its strengths and providing practical examples.
Understanding Blockchain and Decentralized Applications
Blockchain is a distributed ledger technology that ensures data integrity through a chain of blocks. Decentralized applications (dApps) leverage blockchain to operate without a central authority, providing transparency and security. Building dApps requires robust, scalable, and fault-tolerant systems, areas where Elixir excels.
Using Elixir for Blockchain Development
Elixir offers concurrency, fault tolerance, and distributed computing features that make it an excellent choice for blockchain development. Below, we delve into key aspects of using Elixir for blockchain applications, including examples and best practices.
1. Setting Up an Elixir Blockchain Environment
Before diving into blockchain development, setting up your Elixir environment is crucial. This involves installing Elixir, setting up a project, and configuring necessary dependencies.
Example: Setting Up an Elixir Project
```bash mix new blockchain_app --module BlockchainApp cd blockchain_app ```
Add required dependencies to your `mix.exs` file, such as libraries for interacting with blockchain networks.
2. Implementing Basic Blockchain Concepts
To understand how Elixir handles blockchain concepts, let’s implement a simple blockchain that stores and validates transactions.
Example: Creating a Basic Blockchain
```elixir defmodule Blockchain do defstruct [:index, :previous_hash, :timestamp, :data, :hash] @doc """ Creates a new block with given data and previous hash. """ def create_block(data, previous_hash) do index = :rand.uniform(1000) timestamp = :os.system_time(:second) hash = :crypto.hash(:sha256, "#{index}#{previous_hash}#{timestamp}#{data}") |> Base.encode16() %Blockchain{ index: index, previous_hash: previous_hash, timestamp: timestamp, data: data, hash: hash } end end ```
3. Handling Transactions and Smart Contracts
For more advanced blockchain features, such as smart contracts, Elixir’s concurrency model and fault tolerance can be advantageous. Implementing smart contracts in Elixir requires defining transaction processing and validation logic.
Example: Processing Transactions
```elixir defmodule TransactionProcessor do def process_transaction(transaction) do # Transaction processing logic here IO.puts("Processing transaction: #{inspect(transaction)}") end end ```
4. Scaling with Elixir’s Concurrency Model
Elixir’s lightweight processes and message-passing concurrency model make it ideal for scaling blockchain applications. Utilize these features to handle multiple transactions and nodes efficiently.
Example: Concurrency with Elixir
```elixir defmodule Node do def start do spawn(fn -> loop() end) end defp loop do receive do {message, from} -> IO.puts("Received message: #{message}") send(from, "Acknowledged") loop() end end end ```
5. Integrating with Existing Blockchain Networks
Elixir can interact with existing blockchain networks through APIs and libraries. This integration allows for leveraging blockchain features while maintaining Elixir’s advantages.
Example: Fetching Blockchain Data
```elixir defmodule BlockchainAPI do use HTTPoison.Base def fetch_latest_block do get!("https://api.blockchain.com/latest_block") |> Map.get(:body) end end ```
Conclusion
Elixir’s features make it a powerful choice for building decentralized applications on the blockchain. Its concurrency, fault tolerance, and scalability support efficient and reliable blockchain solutions. By harnessing Elixir’s strengths, developers can create robust dApps that leverage the benefits of blockchain technology.
Further Reading:
Table of Contents
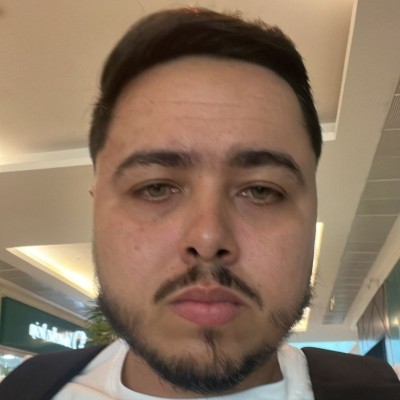
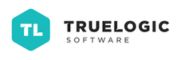