Building a Blog Engine with Elixir and Phoenix
In the world of web development, creating a blog engine is a common task that showcases a developer’s ability to handle data, user authentication, and content management. While there are numerous frameworks and technologies available for this purpose, Elixir and Phoenix offer a unique combination of performance, concurrency, and maintainability. In this tutorial, we’ll take you through the process of building a robust blog engine using Elixir and the Phoenix framework.
Table of Contents
1. Introduction to Elixir and Phoenix
1.1. Brief Overview of Elixir
Elixir is a functional programming language that runs on the Erlang VM, known for its exceptional concurrency handling and fault tolerance. Elixir’s syntax is elegant and expressive, making it an excellent choice for building highly performant applications that can handle numerous concurrent connections.
1.2. Advantages of Using Phoenix for Web Development
Phoenix is a web framework built with Elixir that follows the Model-View-Controller (MVC) pattern. It offers a unique “LiveView” feature, enabling real-time, interactive web applications without the need for complex JavaScript frameworks. Phoenix leverages Elixir’s concurrency model, allowing it to handle thousands of connections simultaneously while maintaining low latency.
2. Setting Up the Project
2.1. Installing Elixir and Phoenix
Before you start building your blog engine, you need to install Elixir and Phoenix. You can find installation instructions on their respective official websites. Once installed, you can use the mix command-line tool to manage your Elixir projects.
2.2. Creating a New Phoenix Project
To create a new Phoenix project, open your terminal and run the following command:
bash mix phx.new BlogEngine --no-ecto --no-webpack
This command creates a new Phoenix project named “BlogEngine” without Ecto (for now) and without the Webpack asset pipeline.
2.3. Project Structure Overview
The generated Phoenix project follows a conventional directory structure. Here are some key directories and their purposes:
- config: Contains configuration files for various environments.
- lib: Houses the Elixir code for your application.
- web: Contains the web-related components, including controllers, views, templates, and LiveView components.
- test: Holds your application’s test files.
- priv: Stores private assets like static files and compiled assets.
In the next section, we’ll delve into building the data model for our blog engine.
3. Building the Data Model
3.1. Designing the Blog Schema
A blog typically consists of posts, categories, and tags. Let’s start by designing the schema for our blog engine using Ecto.
First, create a new context for your blog-related functionality:
elixir mix phx.gen.context Blog Post posts title:string content:text category_id:integer mix phx.gen.context Blog Category categories name:string mix phx.gen.context Blog Tag tags name:string
This will generate Ecto schemas, migrations, and context modules for posts, categories, and tags.
3.2. Using Ecto for Database Interactions
Ecto is Elixir’s database wrapper and query generator. With Ecto, you can perform database operations in a structured and functional way. Create and migrate the database tables by running:
bash mix ecto.create mix ecto.migrate
3.3. Creating Migrations for Posts and Categories
Migrations are scripts that help manage database changes over time. You can find your generated migrations in the priv/repo/migrations directory. Customize these migrations to define your table structures, relationships, and indexes.
In your create_posts.exs migration file, you can define the posts table:
elixir def change do create table(:posts) do add :title, :string add :content, :text add :category_id, references(:categories) timestamps() end end
Similarly, define the categories and tags tables in their respective migration files.
In the next section, we’ll implement user authentication to secure access to the admin panel and blog management functionalities.
4. Implementing User Authentication
4.1. Setting Up User Accounts
Phoenix provides a powerful authentication library called Guardian that can be used to manage user authentication and authorization. Begin by adding Guardian as a dependency in your mix.exs file:
elixir defp deps do [ # ... {:guardian, "~> 2.0"} ] end
Run mix deps.get to fetch the new dependency.
4.2. Using Comeonin for Password Hashing
To securely store user passwords, we’ll use the Comeonin library. Add it as a dependency in your mix.exs:
elixir defp deps do [ # ... {:comeonin, "~> 5.1"} ] end
After fetching the dependency, follow the Comeonin documentation to configure password hashing in your application.
4.3. Securing Routes and Actions
Phoenix uses a concept called plugs to manage middleware-like functionality. Use plugs to secure your routes and actions by authenticating users before granting access. Here’s a simple example of a plug that checks if a user is authenticated:
elixir defmodule BlogEngineWeb.Plugs.Authenticated do import Plug.Conn def init(_opts), do: %{} def call(conn, _opts) do if Guardian.Plug.authenticated?(conn) do conn else conn |> put_flash(:error, "You must be logged in to access this page.") |> redirect(to: Routes.user_session_path(conn, :new)) |> halt() end end end
Apply this plug to your routes or controller actions that require authentication. For instance, you can use it in your admin-related routes.
5. Creating the Blog Interface
Designing the User Interface with HTML and CSS
The user interface of your blog engine can be built using HTML and CSS. Phoenix provides layouts, templates, and views to structure your UI components. You can use front-end libraries like Tailwind CSS or Bulma to style your application.
5.1. Displaying a List of Blog Posts
In your Phoenix view, you can create a function that fetches and displays a list of blog posts. Here’s a simplified example:
elixir defmodule BlogEngineWeb.PostView do use BlogEngineWeb, :view def list_posts(posts) do render_many(posts, __MODULE__, "post.html") end end
In your corresponding template (post.html), you can iterate through the list of posts and display their titles and content.
5.2. Creating and Editing Blog Posts
Building the forms for creating and editing blog posts involves using Phoenix’s form helpers. Define a form in your template, and handle form submissions in your controller. Don’t forget to apply authentication checks to ensure only authorized users can create and edit posts.
5.3. Managing Categories and Tags
Categories and tags can be managed similarly to posts. Create forms for adding new categories and tags, and handle their submissions in your controller. You can then associate categories and tags with blog posts using their respective IDs.
Conclusion
In this tutorial, we’ve explored the process of building a blog engine using Elixir and the Phoenix framework. We’ve covered setting up the project, designing the data model, implementing user authentication, creating the blog interface, and enabling additional features like rich text editing, comments, and pagination. By following these steps and harnessing the power of Elixir and Phoenix, you can create a performant and feature-rich blog engine that showcases your skills as a web developer. Happy coding!
Table of Contents
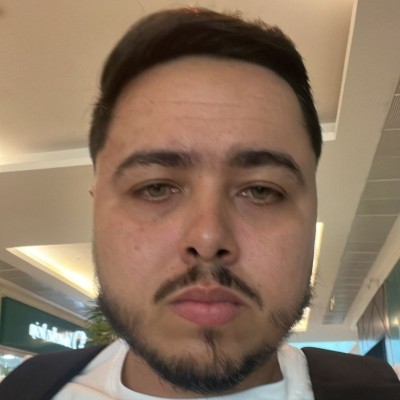
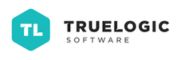